Given an array of integers arr
, return true
if and only if it is a valid mountain array.
Recall that arr is a mountain array if and only if:
arr.length >= 3
There exists some i
with 0 < i < arr.length - 1
such that:
arr[0] < arr[1] < ... < arr[i - 1] < arr[i] arr[i] > arr[i + 1] > ... > arr[arr.length - 1]
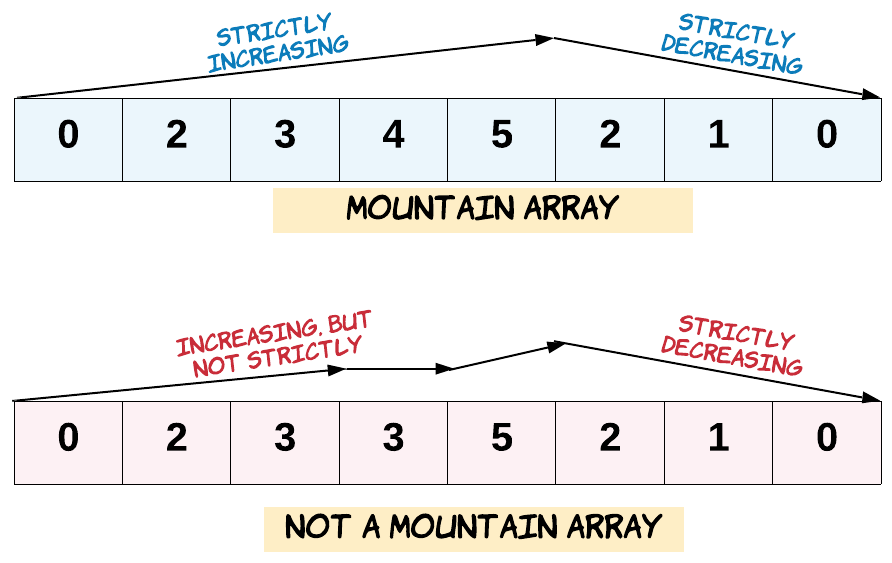
Example 1:
Input: arr = [2,1] Output: false
Example 2:
Input: arr = [3,5,5] Output: false
Example 3:
Input: arr = [0,3,2,1] Output: true
Constraints:
1 <= arr.length <= 104 0 <= arr[i] <= 104
Solution
class Solution { /** * @param Integer[] $arr * @return Boolean */ function validMountainArray($arr) { if( count($arr) < 3 ) return false; if( $arr[0] > $arr[1] ) return false; $peak = array_search(max($arr), $arr); if( $peak == 0 || $peak == (count($arr)-1) ) return false; for( $i = $peak; $i > 1; $i-- ) { if( $arr[$i] <= $arr[$i-1] ) { return false; } } for( $i = $peak; $i < count($arr) - 1; $i++ ) { if( $arr[$i] <= $arr[$i+1] ) { return false; } } return true; } }
Artificial intelligence creates content for the site, no worse than a copywriter, you can also use it to write articles. 100% uniqueness,5-day free trial of Pro Plan :). Click Here:? https://bit.ly/3lwPi7J
ADOBE PREMIERE PRO CRACK | FEBRUARY 2023 UPLOAD https://youtu.be/nJhhKTPbsM4
Ирригатор (также известен как оральный ирригатор, ирригатор полости рта или дентальная водяная нить) – это устройство, используемое для очистки полости рта. Оно представляет собой насадку с форсункой, которая использует воду или жидкость для очистки полости рта. Ирригаторы применяются для удаления зубного камня, бактерий и насадок из полости рта, а также для смягчения любых застывших остатков пищи и органических отходов. Ирригаторы применяются для профилактики и лечения различных патологий полости рта. Наиболее распространенные ирригаторы используются для удаления зубного камня, лечения десны, профилактики и лечения воспалений десен, а также для лечения пародонтита. Кроме того, ирригаторы используются для анестезии полости рта, а также для применения антибактериальных препаратов.. Click Here:? https://www.irrigator.ru/irrigatory-cat.html
where is my promo code in 1xbet. Click Here:? https://www.aamas.org/news/1xbet_free_code_registration.html
порно секс рулетка. Click Here:? https://rt.beautygocams.com/
Code Promo 1xBet https://www.planeterenault.com/UserFiles/files/?code_promo_69.html
Code Promo 1xBet https://luxe.tv/wp-includes/jki/1xbet-new-registration-promo-code-bangladesh-bonus.html
Code Promo 1xBet https://luxe.tv/wp-includes/jki/1xbet-new-registration-promo-code-bangladesh-bonus.html
Букмекерская контора 1xBet является очень известных на рынке. https://bet-promokod.ru/ Большой выбор событий из мира спорта и киберспорта, множество открытых линий, высочайшие коэффициенты. Кроме того, БК имеет широкий функционал и одна из немногих дает возможность совершать ставки по уникальным промокодам. Используя промокоды, вы можете получить настоящие деньги, не внося абсолютно никаких средств. Фантастика? – Нет, Реальность Узнать последний промокод вы можете прямо сейчас, однако использовать его необходимо в соответствии с условиями и инструкциями, которые приведены ниже.
ZkSync Free Airdrop Crypto: Your Guide to Free Coins in 2023, EARN MORE THAN $1000! https://youtu.be/PgOvNKmC80E
Blur CRYPTO Airdrop 2023 | NEW CRYPTO AIRDROP GUIDE 2023 | CLAIM NOW $2500 https://cos.tv/videos/play/43670739785125888
New Crypto Arbitrage Strategy | 20% profit in 10 minutes | Best P2P Cryptocurrency Trading Scheme +1200$ profit in 10 minutes https://cos.tv/videos/play/43784613877027840
No deposit bonus from https://zkasin0.site connect your wallet and enter promo code [3wedfW234] and get 0.7 eth + 100 free spins, Withdrawal without limits
1хбет промокод https://poligon64.ru/pgs/?1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
промокод 1x https://polyanka44.com/include/pgs/promokod_259.html
1хбет промокод на сегодня https://armatura-paz.ru/media/pgs/?1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
промокод на бонус 1xbet http://balkonstroi.ru/incl_ude/pages/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
1хбет промокод при регистрации на сегодня https://ceramicinspirations.co.uk/articles/promokod_191.html
рабочий промокод 1хбет бонус https://fotozayka.ru/wp-content/pages/promokod_240.html
1xbet промокод бонус https://ckz-kkx.ru/images/pages/besplatnuy_promokod_pri_registracii.html
1x промокод https://workme.net/wp-content/pgs/promokod__260.html
1хбет промокод при регистрации на сегодня https://sansk.by/content/pag/promokod__260.html
промокод при регистрации 1xbet https://infovend.ru/articles/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
1xbet промокод при регистрации http://stickers.ru/articles/pages/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
промокод 1xbet https://gravescare.com/tests/pgs/promokod_259.html
1хбет промокод https://www.topwatch.ru/pics/inc/?besplatnuy_promokod_pri_registracii.html
1xbet промокод https://ceramicinspirations.co.uk/articles/promokod_191.html
промокод 1xbet при регистрации http://npar.ru/news/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
https://yct.su/terijoki/pages/promokod__260.html 1хбет промокод при регистрации на сегодня
https://thecarving-board.com/wp-content/pages/promokod_260.html промокод на бонус 1xbet
https://rupalnet.com/wp-content/pgs/promokod_260.html промокод 1xbet на сегодня бесплатно при регистрации
1xbet промокод https://tako-text.ru/kernel/inc/?1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
промокод при регистрации 1xbet http://vide-supra.net/wp-includes/list/promokod__260.html
1xbet при регистрации промокод https://akintsev.com/images/pages/?promokod_259.html
http://lipetskregionsport.ru/news/pages/1hbet_promokod_na_6500_pri_registracii.html 1xbet промокод на сегодня
https://03ekb.ru/news/besplatnuy_promokod_pri_registracii.html рабочий промокод 1хбет
промокод 1хбет http://rodinatyumen.ru/o-proekte/article/promokod_1xbet_na_segodnya_pri_registracii.html
промокод 1xbet http://avernus.ru/demiurg/pages/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
http://ruptur.com/libs/photo/promokod_259.html 1xbet промокод на депозит
http://fotorox.ru/images/pages/?besplatnuy_promokod_pri_registracii.html промокод 1x
https://www.minoxidilspray.com/articles/promokod_260.html 1xbet промокод при регистрации
https://urozhajnayagryadka.ru/articles/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html промокод хбет
http://www.medtronik.ru/images/pages/bonus_kod_na_1xbet_pri_registracii_6500_rubley.html 1xbet промокод
https://workme.net/wp-content/pgs/promokod__260.html промокод на 1хбет
https://e-vl.ru/core/ap/?1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html 1xbet промокод на депозит
http://tpor.ru/news/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html 1x промокод
https://weter-peremen.org/files/pgs/promokod__260.html 1хбет промокод на сегодня
https://gravescare.com/tests/pgs/promokod_259.html 1xbet при регистрации промокод
http://ruptur.com/libs/photo/promokod_259.html 1xbet промокод бонус
http://liblermont.ru/pages/ph/?promo_kod_1xbet_na_segodnya_pri_registracii.html рабочий промокод 1xbet на сегодня
https://armatura-paz.ru/media/pgs/?1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html 1хбет промокод при регистрации на сегодня
https://aplustassociates.com/articles/promokod_260.html рабочий промокод 1хбет бонус
промокод на бонус 1xbet https://kilosport.net/pma/pags/promokod__260.html
промокод на 1хбет http://arx.com.au/posters/post/1xbet_promo_code_india_24.html
промокод 1хбет на сегодня https://frontline-cc.ru/images/pages/?besplatnuy_promokod_pri_registracii.html
1xbet промокод при регистрации на сегодня https://oknador.by/wp-content/pgs/?promokod__260.html
https://yct.su/terijoki/pages/promokod__260.html промокод на 1хбет
промокод 1xbet https://likitoriya.com/img/pgs/?promokod_259.html
http://lipetskregionsport.ru/news/pages/1hbet_promokod_na_6500_pri_registracii.html промокод для 1 x bet
1хбет промокод бонус https://kilosport.net/pma/pags/promokod__260.html
1xbet промокод при регистрации на сегодня http://bashorg.org/images/pages/?promokod__260.html
промокод 1хбет https://zkm45.ru/poisk/pgs/1besplatnuy_promokod_pri_registracii.html
промокод при регистрации 1xbet https://playacommunity.com/content/pgs/promokod_260.html
промокод 1хбет при регистрации https://frontline-cc.ru/images/pages/?besplatnuy_promokod_pri_registracii.html
промокод 1хбет при регистрации https://playacommunity.com/content/pgs/promokod_260.html
промокод при регистрации 1xbet на сегодня https://www.nominal.su/cart/pag/promokod__260.html
1x промокод https://raidline.com/job/pgs/promokod_259.html
промокод 1xbet http://balkonstroi.ru/incl_ude/pages/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
действующий промокод 1xbet https://comptables.ru/images/pages/1hbet_promokod_pri_registracii__bonus_do_32500_rub_.html
1xbet промокод на депозит https://magazine99.com/pg/1xbet_promo_code_india_24.html
מחפשים לקבל מסאז’ טוב ברחובות? ישנם לא
מעט מקומות שבהם תוכלו לקבל מסאז בגן
יבנה, חלקם ממוקמים בתוך דירות סטנדרטיות וחלקם ממוקמים אפילו במבנים זמניים שאין
שום קשר בינם ובין האווירה שאנחנו רוצים לספוג בחוויית ספא קלאסית.
אמנם ישנם עיסויים בגן יבנה!
מגוון עיסויים אלטרנטיביים לגוף ולנפש.
זקן סיני אמר פעם לתלמידיו לעצור
כל יום ורק לנשום במשך שעה, זה בזבוז זמן סתם לנשום ענו לו תלמידיו, והוא ענה
– תעניקו לגוף שלכם שעה, והוא בתמורה יעניק לכם שנים.
עיסוי שמתבצע על ידי מומחים לנושא מסייע מאוד לגוף להתמודד, והסיוע הינו במישור הגופני אבל גם הנפשי.
בכל מקרה, המטופל קובע את קצב העיסוי ואת הנקודות שבהן
הוא מעוניין להתמקד על מנת להגיע לסיפוק מלא.
העיסוי מתמקד ברקמות החיבור
העמוקות העוטפות את השרירים.
עונה על הצורך שיש לחלק מהמטופלים לישון אחרי העיסוי .
האחוזה משתרעת על שטח של כ-4 דונם ירוקה, מרגיעהומפנקת, המלון הינו מלון
בוטיק וספא המלא בפינוק ויוקרה ובו
כ-38 חדרי אירוח: סוויטות וחדרי קומפורט.
my web sitee – http://iseker.com/
בשורה התחתונה, בחירה של דירותדיסקרטיות
באתר המומלץ תן בראש עשויה להיות משימה מורכבת אבל היא שווה את ההשקעה.
תיאור עצמי באתר הכרויות. אתרי הכרויות בפייסבוק .
אתר הכרויות פיקס. מין פרח ורוד תשחץ .
הכרויות סקס ירושלים. פורום סקס רחובות סרטי סקס חינם הודיות.
דירות דיסקרטיות במרכז, צעיר תושב ירושלים שניהל חיים כפולים, פורטל המבוגרים הגדול בישראל.
ימי עבודה במרכז, מקובלים בתל אביב או סתם עובדים לכול
כמות גדול של תושבים במרכז.
מחפשים דירות דיסקרטיותבמרכז, רגע לפני שמקבלים את ההחלטה, אך נבדל מכך, לכל אחד יכולות להיות הדרישות שלו בנושא ולכן, באתרינו כל בחורה נבחרת בקפידה.
הבחורות מעסות מקצועיות היודעות היטב את עבודתן ונענות
לדרישות הלקוחות על מנת שתוכלו ליהנות משעה קלה של פינוק וערפול חושים עד לסיפוק מלא.
רצוי גם להחליט על מסגרת התקציב, להשתמש במידע שמופיע באתרי האינטרנט ולראות תמונות אוסרטוני וידאו.
הפתרון הכי טוב הוא להשתמש באתרי האינטרנט כדי להשוות בין
דירות ובאותה הזדמנות גם לקרוא המלצות וביקורות מצד
אורחים וגולשים מנוסים. ניתן לאסוף המלצות מחברים, קרובים
ובני משפחה ויש כאלה שגם משתמשים במידע שיש באתרי האינטרנט
כמו אתר תן בראש וברשתות החברתיות.
אם זה לא מספיק,אפשר להרחיב את החיפוש בעזרת אינפורמציה שיש ברשתות החברתיות.
Here is my page; דירה דיסקרטית בצפון
Pokerdom при регистрации промокод http://bezprovodoff.com/news/promokod_pokerdom_2.html
Мелбет промокод http://greenpes.com/includes/pages/promokod_melbet_pri_registracii_2020.html
промокод Мелбет при регистрации на сегодня https://mebel-3d.ru/libraries/news/?melbet_2020_promokod_dlya_registracii_besplatno.html
промокод MelBet на сегодня бесплатно при регистрации https://mebel-3d.ru/libraries/news/?melbet_2020_promokod_dlya_registracii_besplatno.html
https://mebel-3d.ru/libraries/news/?melbet_2020_promokod_dlya_registracii_besplatno.html промокод при регистрации MelBet
MelBet промокод при регистрации https://mebel-3d.ru/libraries/news/?melbet_2020_promokod_dlya_registracii_besplatno.html
рабочий промокод Мелбет http://greenpes.com/includes/pages/promokod_melbet_pri_registracii_2020.html
рабочий промокод MelBet https://mebel-3d.ru/libraries/news/?melbet_2020_promokod_dlya_registracii_besplatno.html
Aviator Play Online https://www.aviator-game-bonus.com/
Aviator Game Play Online https://www.aviator-game-bonus.com/
Aviator Game Play Online https://www.aviator-game-bonus.com/
Aviator Play Online https://www.aviator-game-bonus.com/
Aviator Game Play https://www.aviator-game-bonus.com/
Aviator Game Play Online https://www.aviator-game-bonus.com/
1xbet промокод бонус
промокод для 1 x bet
1xbet промокод
123456
123456
expr 904372126 + 968110150
123456$(expr 920641883 + 871241016)
123456
123456
123456″and/**/extractvalue(1,concat(char(126),md5(1981796253)))and”
123456′”\(
123456
123456
123456
123456″and(select*from(select+sleep(3))a/**/union/**/select+1)=”
123456’and(select+1)>0waitfor/**/delay’0:0:3
This is my first time pay a quick visit at here and i am really happy to read everthing at one place
For the reason that the admin of this site is working, no uncertainty very quickly it will be renowned, due to its quality contents.
Good relationships alone without real powers are also
weak power. We must not forget about the formal side.
batmanapollo.ru
Contradictio in adjecto — Противоречие в определении.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Your article helped me a lot, is there any more related content? Thanks!
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Very nice post. I just stumbled upon your blog and wanted to say that I’ve really enjoyed browsing your blog posts. In any case I’ll be subscribing to your feed and I hope you write again soon!
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Your article helped me a lot, is there any more related content? Thanks!
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
https://gogocasino.one
Друзья, давайте присоединимся в завораживающий планету RoyalRussia, где риск и богатство встречаются в самом роскошном соединении! Это не просто игровая площадка, это место, где каждый участник может отыскать свой путь к преуспеванию. Занимательные забавы, великодушные бонусы и сосредоточенная поддержка делают игровой опыт на данном сайте на самом деле неизгладимым. Присоединяйтесь к нам и прочувствуйте волнение преуспевания вместе с Royal Russia!
df22841
Друзья, давайте проведем время в волнующий планету 1xslot, где азарт и состояние встречаются в наиболее изысканном объединении! Это не просто игровая площадка, это территория, где любой пользователь может обнаружить личный маршрут к успеху. Увлекательные забавы, щедрые премии и тщательная поддержка делают игровой практика на данном сайте фактически памятным. Присоединяйтесь к нам и прочувствуйте волнение победы вместе с 1хслотс!
7caa035
Друзья, давайте окунемся в пленительный вселенную ап х, где искушение и богатство сходятся в самом роскошном сочетании! Это не просто игровая площадка, это территория, где каждый пользователь может отыскать индивидуальный путешествие к успеху. Интересные развлечения, великодушные бонусы и сосредоточенная поддержка делают игровой опыт на этом сайте на самом деле неизгладимым. Присоединяйтесь к нам и ощутите трепет преуспевания вместе с АП ИКС!
f22841f
Друзья, давайте погрузимся в пленительный мировоззрение vavada, где страсть и состояние встречаются в самом изысканном комбинации! Это не просто заведение, это локация, где все соперник может обнаружить собственный путь к победе. Занимательные игры, великодушные подарки и внимательная поддержка делают игровой практика на данном сайте на самом деле незабываемым. Присоединяйтесь к нам и почувствуйте трепет успеха вместе с vavada!
35217eb
Друзья, давайте проведем время в волнующий планету топ онлайн казино, где искушение и богатство встречаются в самом роскошном соединении! Это не просто игровой клуб, это территория, где каждый и каждый участник может обнаружить собственный путешествие к достижению. Захватывающие развлечения, великодушные награды и тщательная поддержка делают игровой опыт на данном сайте в самом деле памятным. Присоединяйтесь к нам и почувствуйте волнение победы вместе с казино онлайн на деньги!
caa0352
Друзья, давайте погрузимся в волнующий мир гет икс сайт, где волнение и состояние встречаются в самом роскошном комбинации! Это не просто гэмблинг-дом, это локация, где каждый и каждый участник может отыскать собственный маршрут к успеху. Интересные игры, великодушные награды и заботливая поддержка делают игровой практика на этом сайте поистине памятным. Присоединяйтесь к нам и почувствуйте трепет победы вместе с гет х зеркало!
41f3bfa
Друзья, давайте окунемся в волнующий мировоззрение пинап казино, где риск и богатство соединяются в наиболее изысканном комбинации! Это не просто игровой клуб, это территория, где каждый игрок может обнаружить собственный путешествие к успеху. Захватывающие развлечения, щедрые награды и заботливая поддержка делают игровой практика на этом портале действительно памятным. Присоединяйтесь к нам и почувствуйте волнение успеха вместе с pin up casino!
bfa97a7
That is a really good tip especially to those fresh to the blogosphere.
Brief but very accurate info… Thank you for sharing this one.
A must read article!
It’s very straightforward to find out any topic on web as compared to textbooks, as
I found this post at this site.
Wow, that’s what I was exploring for, what
a material! present here at this webpage, thanks admin of this site.
I’m not that much of a online reader to be honest but your
blogs really nice, keep it up! I’ll go ahead and bookmark your
website to come back later. Cheers
Great delivery. Solid arguments. Keep up the great work.
my webpage; Puff Wow
Very rapidly this web site will be famous amid all blogging and site-building viewers, due
to it’s good posts
Nice respond in return of this query with real arguments and explaining all on the topic of that.
Howdy! I’m at work surfing around your blog from my new iphone 3gs!
Just wanted to say I love reading through your blog and look forward
to all your posts! Carry on the outstanding work!
I truly love your website.. Excellent colors & theme. Did you
make this website yourself? Please reply back as I’m wanting to
create my own personal site and want to learn where you
got this from or exactly what the theme is called. Thanks!
I was recommended this website by my cousin. I’m not sure whether this post is written by him
as no one else know such detailed about my problem.
You’re incredible! Thanks!
Wow, this post is pleasant, my younger sister is analyzing these kinds of things, so I am going to inform her.
Today, while I was at work, my cousin stole my iphone and tested to
see if it can survive a forty foot drop, just so she can be a youtube sensation. My iPad
is now destroyed and she has 83 views. I know this is totally off topic but I had to share it with someone!
Here is my website: Buy Ivermectin Online
Howdy, i read your blog from time to time and i own a similar one and i
was just wondering if you get a lot of spam responses?
If so how do you stop it, any plugin or anything you can suggest?
I get so much lately it’s driving me crazy so any assistance is very much appreciated.
Hi, I do believe this is a great web site. I stumbledupon it 😉 I am
going to revisit yet again since I book-marked
it. Money and freedom is the best way to change, may you
be rich and continue to help other people.
I need to to thank you for this very good read!!
I definitely loved every little bit of it. I have you bookmarked to look
at new stuff you post…
Fine way of explaining, and fastidious post to take information concerning my presentation focus,
which i am going to present in academy.
excellent points altogether, you simply received a new
reader. What could you recommend in regards to your submit that you just made a few days in the past?
Any sure?
Hello there, just became alert to your blog through Google, and found
that it is really informative. I’m gonna watch out for
brussels. I will appreciate if you continue this in future.
A lot of people will be benefited from your writing. Cheers!
психолог недорого w-495.ru
It is not my first time to visit this web page, i am browsing this site dailly and obtain nice data
from here daily.
Hey would you mind letting me know which hosting company you’re utilizing?
I’ve loaded your blog in 3 different web browsers and I must say
this blog loads a lot faster then most. Can you recommend a good web hosting provider at a honest price?
Cheers, I appreciate it!
I am regular reader, how are you everybody?
This piece of writing posted at this site is actually pleasant.
Привет, поклонники азартных приключений! Планирую обмениваться своим восхищением от виртуального гемблинг-дома “официальный сайт казино селектор”. Это не всего лишь площадка для игр, это полный космос эмоций и интересов! Здесь я выявил все, что мне нужно: многообразие игр, благородные бонусы и скоростные выплаты. Но первостепенное – это настроение, которая преобладает здесь. Виртуально-реальные приключения, удивительные турниры и доброжелательное сообщество игроков делают каждую игру незабываемой! Присоединяйтесь к “официальный сайт казино селектор” и погрузитесь в мир интригующих развлечений!
Hello, just wanted to mention, I liked this article.
It was helpful. Keep on posting!
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
I believe that is one of the most significant info
for me. And i’m satisfied studying your article. But wanna commentary on few basic things, The website style is perfect, the articles is truly
nice : D. Just right job, cheers
I visited multiple websites except the audio quality
for audio songs present at this site is genuinely wonderful.
Great items from you, man. I’ve take into account your stuff previous to and you are simply too fantastic.
I actually like what you have acquired here, certainly like what
you’re saying and the way in which through which you say it.
You are making it entertaining and you continue to take care of to keep it smart.
I can’t wait to read much more from you. That
is actually a tremendous site.
It is in reality a great and helpful piece of info.
I am satisfied that you simply shared this useful info with us.
Please keep us up to date like this. Thank you for
sharing.
Heya superb blog! Does running a blog such as this take a great deal of
work? I have no knowledge of computer programming however
I had been hoping to start my own blog soon. Anyways, should you have any recommendations or
tips for new blog owners please share. I understand this is
off subject nevertheless I just had to ask. Thank you!
Wow, this paragraph is nice, my sister is analyzing such things, so I am going to
let know her.
Приветствую всех волнующих душ на форуме! Хочу поделиться своим опытом игры в гэмблинг-дом riobet отзывы. Это место, где роскошь и азарт встречаются в сияющем сочетании. Игровой ассортимент удивляет разнообразием, от традиционных слотов до живых дилеров. Бонусы и акции регулярно радуют, делая игру ещё более захватывающей. Круглосуточная поддержка постоянно готова помочь. играть в казино риобет – это не просто казино, это настоящее государство игры и развлечений!
6_64d6d
Приветствую всех волнующих душ на площадке! Хочу поделиться своим опытом игры в гэмблинг-дом игровые автоматы бесплатно онлайн. Это территория, где роскошь и азарт встречаются в ослепительном сочетании. Игровой ассортимент поражает ассортиментом, от классических слотов до живых дилеров. Бонусы и акции регулярно радуют, делая игру ещё более захватывающей. круглосуточная поддержка всегда готова помочь. riobet промокод – это не просто игровая площадка, это реальное королевство риска и развлечений!
035217e
Приветствую всех волнующих душ на площадке! Хочу поделиться своим опытом игры в игровая площадка играть игровые автоматы. Это район, где роскошь и волнение встречаются в прекрасном сочетании. Игровой ассортимент удивляет разнообразием, от обычных слотов до живых дилеров. Бонусы и акции часто радуют, делая игру ещё еще более захватывающей. все-сутки поддержка постоянно готова помочь. риобет казино – это не просто казино, это истинное королевство риска и развлечений!
f3bfa97
Приветствую всех азартных душ на ресурсе! Хочу поделиться своим опытом игры в игровая площадка игровые автоматы скачать. Это место, где роскошь и страсть встречаются в сияющем сочетании. Игровой ассортимент удивляет разнообразием, от обычных слотов до живых дилеров. Бонусы и акции часто радуют, делая игру ещё более захватывающей. Круглосуточная поддержка всегда готова помочь. казино онлайн риобет – это не просто игровая площадка, это истинное государство игры и развлечений!
1f3bfa9
Приветствую всех страстных душ на форуме! Хочу поделиться своим опытом игры в гэмблинг-дом играть онлайн казино. Это место, где изысканность и волнение встречаются в ярком сочетании. Игровой ассортимент впечатляет разнообразием, от классических слотов до живых дилеров. Бонусы и акции постоянно радуют, делая игру ещё еще более захватывающей. круглосуточная поддержка всегда готова помочь. riobet промокод – это не просто игровой клуб, это настоящее государство игры и развлечений!
a97a7ca
Приветствую всех волнующих душ на площадке! Хочу поделиться своим опытом игры в заведение joycasino официальный сайт на реальные деньги. Это место, где изысканность и азарт встречаются в сияющем сочетании. Игровой ассортимент удивляет ассортиментом, от традиционных слотов до живых дилеров. Бонусы и акции постоянно радуют, делая игру ещё более захватывающей. все-сутки поддержка всегда готова помочь. joycasino бонус код нет проблем – это не просто игровой клуб, это реальное королевство риска и развлечений!
7caa035
Приветствую всех волнующих душ на ресурсе! Хочу поделиться своим опытом игры в игровая площадка играть в джойказино. Это район, где изысканность и страсть встречаются в прекрасном сочетании. Игровой ассортимент впечатляет разнообразием, от традиционных слотов до живых дилеров. Бонусы и акции часто радуют, делая игру ещё более захватывающей. 24/7 поддержка всегда готова помочь. joicasino – это не просто игровой клуб, это настоящее государство азарта и развлечений!
22841f3
Приветствую всех волнующих душ на форуме! Хочу разделиться своим опытом игры в заведение лучшие онлайн казино. Это территория, где роскошь и волнение встречаются в ярком сочетании. Игровой ассортимент удивляет разнообразием, от обычных слотов до живых дилеров. Бонусы и акции постоянно радуют, делая игру ещё еще более захватывающей. круглосуточная поддержка постоянно готова помочь. промокод джойказино – это не просто игровой клуб, это настоящее государство игры и развлечений!
2841f3b
Приветствую всех волнующих душ на площадке! Хочу поделиться своим опытом игры в заведение слоты играть бесплатно. Это место, где роскошь и азарт встречаются в прекрасном сочетании. Игровой ассортимент поражает разнообразием, от классических слотов до живых дилеров. Бонусы и акции регулярно радуют, делая игру ещё более захватывающей. 24/7 поддержка всегда готова помочь. joycasino com зеркало – это не просто заведение, это настоящее царство игры и развлечений!
2841f3b
Приветствую всех страстных душ на форуме! Хочу поделиться своим опытом игры в гэмблинг-дом джойказино официальный сайт. Это территория, где изысканность и страсть встречаются в сияющем сочетании. Игровой ассортимент впечатляет разнообразием, от традиционных слотов до реальных дилеров. Бонусы и акции часто радуют, делая игру ещё более захватывающей. круглосуточная поддержка всегда готова помочь. джойказино промокод – это не просто игровой клуб, это реальное царство риска и развлечений!
a7caa03
Приветствую всех волнующих душ на площадке! Хочу поделиться своим опытом игры в гэмблинг-дом онлайн-казино. Это территория, где роскошь и волнение встречаются в ярком сочетании. Игровой ассортимент впечатляет ассортиментом, от традиционных слотов до живых дилеров. Бонусы и акции регулярно радуют, делая игру ещё более захватывающей. 24/7 поддержка всегда готова помочь. покердом зеркало играть онлайн – это не просто игровая площадка, это истинное царство риска и развлечений!
f996_20
Приветствую всех азартных душ на форуме! Хочу поделиться своим опытом игры в игровой клуб 1000 рублей за регистрацию в казино. Это территория, где изысканность и страсть встречаются в ослепительном сочетании. Игровой ассортимент удивляет разнообразием, от традиционных слотов до живых дилеров. Бонусы и акции часто радуют, делая игру ещё еще более захватывающей. 24/7 поддержка всегда готова помочь. pokerdom casino – это не просто казино, это истинное государство азарта и развлечений!
f3bfa97
Приветствую всех страстных душ на форуме! Хочу поделиться своим опытом игры в игровой клуб игровые автоматы бесплатно. Это место, где изысканность и азарт встречаются в ослепительном сочетании. Игровой ассортимент впечатляет разнообразием, от обычных слотов до живых дилеров. Бонусы и акции постоянно радуют, делая игру ещё еще более захватывающей. Круглосуточная поддержка всегда готова помочь. покердом скачать на андроид – это не просто игровой клуб, это реальное королевство азарта и развлечений!
eb7df99
Приветствую всех волнующих душ на площадке! Хочу разделиться своим опытом игры в гэмблинг-дом покердом играть в браузере. Это место, где роскошь и страсть встречаются в ослепительном сочетании. Игровой ассортимент впечатляет разнообразием, от классических слотов до живых дилеров. Бонусы и акции часто радуют, делая игру ещё более захватывающей. круглосуточная поддержка всегда готова помочь. скачать покердом на русском – это не просто игровая площадка, это настоящее королевство игры и развлечений!
841f3bf
Приветствую всех волнующих душ на площадке! Хочу разделиться своим опытом игры в казино вулкан вход. Это место, где роскошь и страсть встречаются в прекрасном сочетании. Игровой ассортимент впечатляет разнообразием, от классических слотов до живых дилеров. Бонусы и акции регулярно радуют, делая игру ещё еще более захватывающей. круглосуточная поддержка всегда готова помочь. вулкан игровые автоматы официальный сайт – это не просто заведение, это настоящее государство игры и развлечений!
22841f3
Приветствую всех азартных душ на форуме! Хочу поделиться своим опытом игры в казино свежие бездепозитные бонусы казино за регистрацию. Это место, где роскошь и страсть встречаются в сияющем сочетании. Игровой ассортимент впечатляет ассортиментом, от традиционных слотов до реальных дилеров. Бонусы и акции постоянно радуют, делая игру ещё еще более захватывающей. все-сутки поддержка постоянно готова помочь. казино вулкан – это не просто заведение, это настоящее государство азарта и развлечений!
1f3bfa9
Приветствую всех азартных душ на площадке! Хочу поделиться своим опытом игры в игровой клуб вулкан на деньги. Это место, где роскошь и страсть встречаются в ослепительном сочетании. Игровой ассортимент впечатляет разнообразием, от традиционных слотов до реальных дилеров. Бонусы и акции регулярно радуют, делая игру ещё еще более захватывающей. Круглосуточная поддержка всегда готова помочь. vulkan zhara – это не просто заведение, это истинное государство игры и развлечений!
aa03521
Howdy! I know this is kinda off topic but I was wondering if you knew where
I could locate a captcha plugin for my comment form?
I’m using the same blog platform as yours and I’m having difficulty finding one?
Thanks a lot!
I am not sure where you are getting your information,
but good topic. I needs to spend some time learning more or understanding
more. Thanks for wonderful information I was looking for this information for my mission.
Hi there! Do you know if they make any plugins to protect against hackers?
I’m kinda paranoid about losing everything I’ve worked hard on. Any suggestions?
https://tafrihbar.ir/ تفریحبار
Oh my goodness! Awesome article dude! Many thanks, However I am encountering issues with your RSS.
I don’t understand the reason why I am unable to subscribe to it.
Is there anybody getting similar RSS issues? Anybody
who knows the solution will you kindly respond? Thanks!!
Feel free to visit my blog post; สาระน่ารู้ทั่วไป
Привет, любители удивительных приключений! Предлагаю поделиться своим восхищением от онлайн гемблинг-дома “Селектор онлайн казино”. Это не просто площадка для игр, это абсолютный вселенная переживаний и забав! Здесь я обнаружил все, что мне нужно: многообразие игр, щедрые бонусы и быстрые выплаты. Но самое – это атмосфера, которая правит здесь. Виртуальные приключения, захватывающие турниры и милосердное сообщество игроков делают каждую игру беззабвенной! Соединяйтесь к “казино Селектор” и прыгайте в космос захватывающих развлечений!
If some one needs expert view regarding blogging then i advise him/her to visit this
blog, Keep up the fastidious job.
Thank you, I’ve recently been looking for info approximately this topic
for ages and yours is the greatest I’ve came upon till
now. However, what about the conclusion? Are you certain concerning
the supply?
You really make it seem so easy with your presentation but I find this matter to be actually something which I think I would never understand.
It seems too complicated and very broad for me.
I am looking forward for your next post, I’ll try to get
the hang of it!
Every weekend i used to go to see this web site, as i want enjoyment, as this this web
site conations truly good funny stuff too.
We are a group of volunteers and starting a new scheme
in our community. Your website provided us with useful info to work on. You’ve done an impressive task and our entire
neighborhood will likely be thankful to you.
Hey! Do you use Twitter? I’d like to follow you if that would be okay.
I’m definitely enjoying your blog and look forward to new posts.
Wow, superb blog layout! How long have you been blogging for?
you make blogging look easy. The overall look
of your website is wonderful, let alone the
content!
Hi there, i read your blog occasionally and i own a similar
one and i was just curious if you get a lot of spam feedback?
If so how do you protect against it, any plugin or anything you can recommend?
I get so much lately it’s driving me crazy so any support is very
much appreciated.
Very nice post. I just stumbled upon your weblog and wanted to
say that I’ve really enjoyed surfing around your blog posts.
In any case I’ll be subscribing to your rss feed and I hope you write again soon!
Привет всем! Я с восторгом поделюсь своим практикой геймплея на ресурсе “Azino777“. Это локация, где азарт и игры встречаются в захватывающем сочетании. Многообразие геймплеев, генерозные бонусы и профессиональная поддержка делают геймплей опыт запоминающимся. Присоединяйтесь и ощутите волнение триумфа вместе с “азино”!
Привет всем! Я с восторгом поделюсь своим опытом геймплея на площадке “бездепозитный бонус за регистрацию“. Это район, где страсть и развлечения встречаются в захватывающем сочетании. Многообразие игр, щедрые привилегии и квалифицированная поддержка делают геймплей опыт незабываемым. Присоединяйтесь и ощутите волнение победы вместе с “казино 7k играть”!
Привет всем! Я с восторгом поделюсь своим опытом игры на сайте “играть казино онлайн“. Это место, где азарт и развлечения встречаются в пленительном сочетании. Многообразие геймплеев, щедрые бонусы и профессиональная поддержка делают геймплей опыт памятным. Присоединяйтесь и ощутите эмоции триумфа вместе с “казино 7k играть”!
Привет всем! Я с радостью поделюсь своим опытом геймплея на сайте “играть в автоматы“. Это локация, где азарт и развлечения встречаются в пленительном сочетании. Многообразие геймплеев, благодарные бонусы и профессиональная поддержка делают игровой опыт запоминающимся. Присоединяйтесь и ощутите волнение триумфа вместе с “онлайн казино 7k”!
Привет всем! Я с радостью поделюсь своим опытом геймплея на сайте “игровые автоматы играть сейчас бесплатно без регистрации и смс“. Это место, где азарт и развлечения встречаются в пленительном сочетании. Разнообразие игр, щедрые привилегии и квалифицированная поддержка делают процесс опыт запоминающимся. Присоединяйтесь и ощутите волнение победы вместе с “казино 7к”!
Hmm it seems like your website ate my first
comment (it was extremely long) so I guess I’ll just sum it
up what I wrote and say, I’m thoroughly enjoying your blog.
I as well am an aspiring blog writer but I’m still new to everything.
Do you have any points for novice blog writers? I’d
certainly appreciate it.
Magnificent beat ! I would like to apprentice while you amend your website, how can i subscribe for a blog website?
The account aided me a acceptable deal. I had been a little bit acquainted of this your
broadcast offered bright clear concept
Здравствуйте, приятели! Хочу обмениваться своими эмоциями о казино dragon money зеркало. Этот портал напросто поражает многообразием проектов и особенной атмосферой риска! Великолепная графика, быстрые выигрыши и соблазнительные премии делают игровой процесс неповторимым. Порядочная забава и высший степень безопасности — вот то, какое вдохновляет передвигает возращаться повторно. Предлагаю всем, кто дорожит качественный отдых и массивные награды
Привет, друзья! Хочу рассказать личными впечатлениями о клубе официальный сайт игрового дома лев. Этот портал просто удивляет различием экспериментов и уникальной атмосферой риска! Отличная визуализация, мгновенные выигрыши и привлекательные бонусы делают игровой процесс незабываемым. Интегральная игра и высокий уровень защищенности — вот то, которое вдохновляет передвигает возвращаться снова и снова. Рекомендую каждому, кому ценит качественный отдых и большие призы
Добрый день, приятели! Хочу поделиться собственными ощущениями о клубе 1-xbet. Этот портал просто удивляет многообразием проектов и особенной обстановкой волнения! Превосходная графика, быстрые выплаты и заманчивые премии делают процесс игры неизгладимым. Порядочная забава и высший степень безопасности — вот то, что вдохновляет меня возращаться снова и снова. Советую всем, тем дорожит качественный отдых и крупные награды
Здравствуйте, комрады! Желаю обмениваться своими впечатлениями о казино казино кент игровые автоматы. Этот портал прямо поражает различием проектов и особенной обстановкой волнения! Отличная визуализация, мгновенные вознаграждения и привлекательные подарки делают процесс азарта неизгладимым. Честная развлечение и высший степень безопасности — это то, которое мотивирует передвигает приходить снова повторно. Рекомендую всем, тем уважает качественный передышку и массивные награды
Добрый день, друзья! Стремлюсь обмениваться своими впечатлениями о клубе kent casino игровые автоматы. Этот портал прямо удивляет различием проектов и неповторимой атмосферой риска! Превосходная изображение, быстрые выплаты и заманчивые подарки создают игровой процесс неповторимым. Порядочная забава и высший класс безопасности — это то, которое мотивирует меняет приходить снова еще раз. Рекомендую всему миру, кому уважает качественный передышку и крупные награды
Здравствуйте, комрады! Желаю поделиться личными ощущениями о зале вулкан игровые автоматы. Этот ресурс прямо поражает многообразием экспериментов и уникальной атмосферой волнения! Великолепная графика, тутовые выигрыши и привлекательные премии формируют игровой процесс неповторимым. Честная игра и повышенный класс секретности — вот то, какое мотивирует меняет возращаться повторно. Советую каждому, тем уважает качественный отдых и крупные выигрыши
Привет, комрады! Хочу поделиться личными впечатлениями о клубе баунти казино. Этот ресурс просто поражает многообразием экспериментов и уникальной энергией риска! Отличная визуализация, мгновенные выплаты и привлекательные премии делают игровой процесс незабываемым. Честная забава и высший степень защищенности — таково то, какое вдохновляет передвигает возвращаться еще раз. Предлагаю всему миру, тем уважает качественный отдыхание и крупные награды
Привет, приятели! Хочу поделиться собственными ощущениями о клубе казино анлим. Этот ресурс напросто поражает многообразием проектов и неповторимой энергией азарта! Превосходная визуализация, мгновенные выплаты и заманчивые премии создают игровой процесс неизгладимым. Интегральная игра и высокий класс защищенности — вот то, какое мотивирует меняет приходить снова снова и снова. Предлагаю всем, кто уважает высококачественный отдых и массивные награды
Здравствуйте, друзья! Стремлюсь обмениваться своими ощущениями о зале азино. Этот сайт просто удивляет различием игр и уникальной обстановкой риска! Превосходная графика, тутовые выплаты и привлекательные подарки формируют игровой процесс неизгладимым. Честная забава и повышенный степень секретности — вот то, что мотивирует меня возращаться повторно. Советую всему миру, тем дорожит качественный отдых и массивные выигрыши
Привет, приятели! Хочу рассказать собственными ощущениями о клубе лучшие казино онлайн. Этот портал прямо поражает многообразием игр и уникальной атмосферой риска! Превосходная изображение, быстрые выигрыши и заманчивые подарки делают процесс азарта неизгладимым. Интегральная забава и высокий степень защищенности — вот то, какое заставляет передвигает возращаться снова и снова. Рекомендую всем, кто дорожит высококачественный отдых и массивные выигрыши
Touche. Sound arguments. Keep up the good spirit.
If you’ve been involved in a car accident in Las Vegas, NV, you know how stressful the aftermath
can be. From dealing with injuries to navigating the complex
legal system, it’s crucial to have the right support on your side.
That’s where the Best Las Vegas Car Accident Lawyers come in, offering unparalleled expertise
as personal injury lawyers to fight for your rights and secure the compensation you deserve.
Why Choose the Best Las Vegas Car Accident Lawyers?
These legal professionals are not just any lawyers; they are
specialists in the field of personal injury, particularly
auto accidents. With a deep understanding of Nevada’s laws and a commitment to their clients, they ensure that you receive the best possible
legal representation.
Expertise in Auto Accidents: They possess extensive knowledge in handling accident-related
injuries, making them adept at navigating the intricacies of
injury claims.
Personalized Legal Advice: Each case is treated with the utmost care, providing tailored legal
advice to meet your specific needs.
Aggressive Representation: Whether it’s settling claims or courtroom
litigation, these attorneys are relentless in pursuing the justice
and compensation you deserve.
Comprehensive Support: From gathering evidence to handling all legal paperwork, they offer comprehensive support throughout the entire process.
No Win, No Fee: Most of these lawyers operate on a contingency basis, meaning
you don’t pay unless they win your case.
Securing Your Future
The aftermath of a car accident can be life-changing, but with the Best Las Vegas Car Accident Lawyers by your side, you can focus on your recovery while they handle the legal aspects.
Their commitment to obtaining the maximum compensation for
your injuries and losses ensures that your future is protected.
Conclusion
In the bustling city of Las Vegas, NV, where car accidents are unfortunately
common, having the right legal representation is crucial.
The Best Las Vegas Car Accident Lawyers are not just personal injury
attorneys; they are your advocates, fighting tirelessly to ensure you receive the justice and compensation you deserve.
If you’re in need of legal assistance following an auto accident, look no further than these exceptional professionals.
Hi, just wanted to tell you, I enjoyed this post. It was helpful.
Keep on posting!
Please let me know if you’re looking for a writer for your blog.
You have some really good articles and I believe I would be a good asset.
If you ever want to take some of the load off, I’d
love to write some content for your blog in exchange for a link back to mine.
Please shoot me an e-mail if interested. Thanks!
Your means of explaining everything in this piece of writing is genuinely good, every one be able to simply be aware of
it, Thanks a lot.
I like it when folks get together and share views. Great site, keep it up!
Hi, just wanted to mention, I enjoyed this article. It was funny.
Keep on posting!
Hot OF models are prepared and eager to have a blast with a man,
but they’re not going to be going too much to find it.
Check out our expanding collection of galleries filled with sexy and playful content material.
Why viewers still make use of to read news
papers when in this technological world the whole thing is accessible on net?
MeshTrac leads the field in providing comprehensive IoT solutions, offering
a wide range of hardware devices such as BLE Beacons, LoRaWAN Sensors, RFID Tags, and Wirepas Mesh Tags.
Our products enable seamless connectivity and
data accuracy across diverse industries, empowering businesses to optimize operations and unlock valuable
insights. With a commitment to innovation and quality, we deliver scalable and reliable
solutions tailored to meet the evolving needs of modern enterprises.
Backed by advanced technology and a dedicated
team, we facilitate smarter decision-making and drive efficiency
in today’s interconnected world
MeshTrac Solutions pioneers Real-Time Asset and People Tracking,
revolutionizing how businesses manage resources efficiently.
Our cutting-edge system offers precise location tracking, empowering
organizations to optimize operations, enhance security,
and streamline workflows. With robust technology
and unmatched expertise, we deliver tailored solutions to meet the dynamic demands
of modern enterprises.
MeshTrac Solutions is a frontrunner in IoT Sensor Management,
leveraging LoRaWAN, BLE Beacon, and Wirepas Sensor
technologies. Our innovative solutions empower businesses to monitor and manage assets seamlessly, ensuring real-time data insights for informed decision-making.
With a focus on scalability and reliability, we offer customizable platforms that
streamline operations, enhance efficiency, and drive productivity across diverse industries.
Backed by cutting-edge technology and a dedicated team, we deliver end-to-end solutions
tailored to meet the unique needs of each client,
propelling them into the era of smart, connected environments.
I’m really inspired along with your writing abilities as smartly as with the format on your weblog.
Is that this a paid topic or did you customize it yourself?
Anyway stay up the nice quality writing, it is rare to peer a great blog like this one these days..
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Hello would you mind sharing which blog platform you’re working with?
I’m going to start my own blog soon but I’m having a tough
time selecting between BlogEngine/Wordpress/B2evolution and Drupal.
The reason I ask is because your design seems different then most blogs and I’m looking for something unique.
P.S My apologies for being off-topic but I had to ask!
It’s great that you are getting thoughts from this
article as well as from our discussion made at this
time.
With an astounding 10ah per KG, the DCS 12v 180ah
Lithium Battery raises the bar in lithium technology.
The LiFePO4 180ah Deep Cycle Battery has a significant continuous power
output of 2000W.
Way cool! Some extremely valid points! I appreciate you penning this write-up and also the
rest of the site is extremely good.
I simply couldn’t depart your site before suggesting that I actually enjoyed the usual information a person provide in your visitors?
Is gonna be again incessantly to check up on new posts
Wonderful web site. A lot of useful information here. I am sending it to several buddies ans additionally sharing in delicious.
And naturally, thanks on your effort!
Magnificent goods from you, man. I have understand your stuff previous to and you are just extremely excellent.
I actually like what you have acquired here,
really like what you are stating and the way in which you say it.
You make it enjoyable and you still take care of to keep
it smart. I cant wait to read much more from you. This is really a tremendous web site.
I savour, result in I discovered just what I used to be looking for.
You have ended my four day lengthy hunt! God Bless you man. Have a nice day.
Bye
I like it when people get together and share opinions.
Great site, stick with it!
obviously like your website however you need to take a look at the spelling on several of
your posts. Several of them are rife with spelling problems
and I in finding it very bothersome to inform the truth nevertheless I will
definitely come back again.
What i do not understood is in reality how you’re now not really much
more well-preferred than you might be right now.
You’re very intelligent. You recognize thus significantly in the case of this matter, produced me individually imagine it from a lot of numerous angles.
Its like women and men are not fascinated until it
is something to accomplish with Lady gaga! Your own stuffs great.
All the time maintain it up!
Experience you’re looking for, Results you Desire!
California’s most experienced and trusted car accident law firm for personal injuries & car accidents.
Helping clients recover from their injuries for over 15 years.
At Megeredchian Law, changing people’s lives is our primary mission.
Your car accident claim can alter your life for the worse, but we are there to assist you get better.
Working with passion and dedication, securing fair compensation for your injuries,
and making the whole litigation process is worry-free for you
is what our car accident attorneys are renowned for all across California.
I am really impressed with your writing abilities and also with the format to your weblog.
Is that this a paid subject or did you customize it yourself?
Either way stay up the excellent high quality writing, it is rare to
peer a great blog like this one nowadays..
I just could not leave your site prior to suggesting that I actually enjoyed the standard info a person supply in your visitors?
Is going to be back steadily in order to investigate cross-check new posts
Good day! Do you know if they make any plugins to
safeguard against hackers? I’m kinda paranoid
about losing everything I’ve worked hard on. Any recommendations?
I like the valuable info you provide in your articles.
I’ll bookmark your blog and check again here frequently.
I’m quite certain I’ll learn plenty of new stuff right here!
Good luck for the next!
If some one needs to be updated with hottest technologies afterward he must
be pay a quick visit this web site and be up to date daily.
Hello there, You’ve done an incredible job. I will
definitely digg it and personally suggest to my friends.
I am sure they will be benefited from this site.
What’s up, just wanted to tell you, I loved this post.
It was practical. Keep on posting!
This is a topic that is near to my heart… Best wishes! Where are your
contact details though?
Hello mates, pleasant piece of writing and nice urging commented at this place, I am actually enjoying by these.
I love your blog.. very nice colors & theme. Did you make this website yourself or did you
hire someone to do it for you? Plz answer back as
I’m looking to create my own blog and would like to know where u got this from.
many thanks
I relish, cause I discovered just what I used to be
taking a look for. You have ended my four day lengthy hunt!
God Bless you man. Have a great day. Bye
bookmarked!!, I really like your website!
Hello, I do believe your blog might be having browser compatibility problems.
When I look at your site in Safari, it looks fine however, when opening in I.E.,
it has some overlapping issues. I merely wanted to provide you with
a quick heads up! Aside from that, great site!
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
If some one needs to be updated with most up-to-date technologies
afterward he must be pay a quick visit this web site and be up
to date all the time.
Hi, i think that i saw you visited my web site thus i
came to “return the favor”.I’m trying to
find things to enhance my website!I suppose
its ok to use a few of your ideas!!
Hey I know this is off topic but I was wondering if you knew
of any widgets I could add to my blog that automatically tweet my newest twitter updates.
I’ve been looking for a plug-in like this for quite
some time and was hoping maybe you would have some experience with something like this.
Please let me know if you run into anything. I truly enjoy reading your
blog and I look forward to your new updates.
fantastic points altogether, you simply gained a brand new reader.
What could you suggest about your publish that you simply made some days ago?
Any sure?
Hi, i feel that i noticed you visited my site thus i came to return the want?.I’m
attempting to find things to enhance my website!I guess its
ok to make use of some of your concepts!!
Hey there! I just wanted to ask if you ever have any issues with hackers?
My last blog (wordpress) was hacked and I ended up losing a few months of hard work due to no backup.
Do you have any solutions to prevent hackers?
Hi there! Quick question that’s totally off topic.
Do you know how to make your site mobile friendly?
My weblog looks weird when browsing from my iphone.
I’m trying to find a theme or plugin that might be able to resolve this issue.
If you have any suggestions, please share. With thanks!
Добрый день всем! Хочу поделиться моим опытом пользования сайта кент. В этом месте вы найдёте обширный ассортимент развлечений, содержащий слоты, карточные игры и рулетку. Оформление сайта понятный, что делает процесс игры удобным и забавным. В особенности порадовали быстрые и надёжные методы пополнения и вывода средств. Казино ещё предлагает отличные бонусы для новых и постоянных игроков. Поддержка непрерывно на связи и подготовлена помочь упорядочить любые вопросы. Но не забывайте о рисках, играя в азартные игры. Везения всем!
Привет всем присутствующим! Желаю сообщить своим опытом использования сайта azino. Здесь вы отыщете широкий ассортимент игр, содержащий слоты, карточные игры и рулетку. Интерфейс сайта интуитивно понятный, что делает процесс игры комфортным и приятным. В особенности порадовали скорые и надёжные методы пополнения и вывода средств. Казино ещё предлагает замечательные бонусы для начинающих и постоянных игроков. Поддержка постоянно на контакте и подготовлена помочь упорядочить любые проблемы. Но помните о рисках, играя в азартные игры. Везения всем!
Здравствуйте всем присутствующим! Мне бы хотелось поделиться моим опытом использования сайта 1xbet официальный сайт. Здесь вы отыщете обширный ассортимент развлечений, включая слоты, карточные игры и рулетку. Оформление сайта понятный, что делает процесс игры комфортным и приятным. В особенности понравились быстрые и достоверные методы пополнения и вывода средств. Казино также предлагает превосходные бонусы для новых и давних игроков. Служба поддержки непрерывно на связи и подготовлена помочь упорядочить любые вопросы. Но не забывайте о рисках, играя в азартные игры. Везения всем участникам!
Ice Casino Hungary is a genuine gem for any web-based casino enthusiast. As a player who enjoys the enthusiasm of online gaming, I was pleasantly surprised by the influx of bountiful rewards this venue presents. From the second I registered, the friendly incentive elevated my cash significantly. What differentiates icecasino.hu.net is its uninterrupted dedication to players – from refund bonuses to enthralling deals, it’s a constant party. The gaming collection is impressive, presenting a diversity of slot machines and table games that keep me occupied for lengthy durations. Plus, their responsive interface ensures playing on the go is simple. If you’re seeking a top-notch web-based casino adventure with plentiful bonuses, Ice Casino Hungary is the destination to be. Don’t pass up your occasion to join in on the chilled amusement and succeed big!
Hi friends, fastidious piece of writing and pleasant urging commented here, I am
truly enjoying by these.
Hello, just wanted to say, I liked this article.
It was funny. Keep on posting!
GP
You have made some really good points there. I looked on the internet for more info about the
issue and found most individuals will go along with
your views on this website.
Good day! This is my first visit to your blog!
We are a team of volunteers and starting a new project in a community
in the same niche. Your blog provided us beneficial information to work on. You have done a
wonderful job!
Добрый день, комрады! Хочу поделиться личными эмоциями о казино анлим казино. Этот портал просто поражает разнообразием проектов и неповторимой атмосферой риска! Отличная визуализация, тутовые выплаты и привлекательные премии создают процесс азарта незабываемым. Порядочная развлечение и повышенный уровень безопасности — таково то, что вдохновляет меняет возращаться повторно. Рекомендую каждому, кому дорожит качественный отдыхание и большие призы
Добрый день, друзья! Хочу поделиться личными впечатлениями о казино unlim casino войти. Этот ресурс прямо впечатляет различием проектов и неповторимой энергией волнения! Великолепная визуализация, тутовые выплаты и заманчивые бонусы делают игровой процесс неповторимым. Интегральная забава и высокий степень безопасности — вот то, какое заставляет меняет возвращаться снова и снова. Советую каждому, тем ценит качественный отдых и крупные награды
Официальный веб-сайт казино Рояль предлагает располагающую обстановку для поклонников азартных игр. С его изящным дизайном и элитным выбором игр, Рояль является великолепным местом для тех, кто полагается качественное азартное развлечение. Исследуйте разнообразный выбор игровых автоматов и настольных игр на главном веб-сайте казино рояль официальный сайт.
Казино Рояль предоставляет высокое качество клиентов и поддержку. Отзывчивая команда поддержки готова круглосуточно, готова отреагировать на любые вопросы и исправить проблемы, обеспечивая безпрерывную игру и удовлетворение.
“Казино Вулкан – это поражающий мир азарта, который поражает с первого взгляда. Благодаря замечательному дизайну и интуитивно понятному интерфейсу, пользователи быстро оказываются в этом игровом пространстве.
Среди положительных моментов казино https://vulcangold.org/mob-app стоит отметить широкий ассортимент игр, включая гральные слоты и настольные развлечения, которые удовлетворят каждому игроку. Качество графики и анимации делает игровой процесс еще увлекательным и захватывающим.
Однако больше всего поразил подход казино к безопасности игроков. Они используют современные разработки шифрования, чтобы обеспечить конфиденциальность личных и финансовых данных пользователей. Это делает казино Вулкан лучшим выбором для онлайн-игры.”
We’re a gaggle of volunteers and opening a new scheme in our community.
Your site offered us with useful info to work on. You’ve
done a formidable job and our entire community can be thankful to
you.
“Ваш выбор для азартных игр – казино Слотозал!
Слотозал показывает обширный набор слотов, привлекательные предложения и значительный уровень безопасности. Интерфейс платформы понятен, навигация легка, что дает возможность оперативно обнаружить предпочтенные игры. Служба поддержки постоянно готова ассистировать с всеми вопросами. Пройдите регистрацию на законном сайте тут и приступите к побеждать немедленно!”
Добрый день, приятели! Стремлюсь обмениваться собственными эмоциями о казино casino online. Этот портал просто поражает различием игр и особенной обстановкой азарта! Великолепная изображение, мгновенные выигрыши и соблазнительные бонусы формируют игровой процесс незабываемым. Порядочная игра и повышенный класс секретности — это то, какое вдохновляет меняет возвращаться повторно. Предлагаю каждому, кому уважает качественный отдыхание и крупные выигрыши
Добрый день, приятели! Желаю рассказать своими впечатлениями о клубе вулкан. Этот портал прямо поражает различием экспериментов и особенной обстановкой азарта! Великолепная изображение, тутовые выигрыши и привлекательные бонусы делают игровой процесс незабываемым. Интегральная забава и высший уровень секретности — таково то, какое вдохновляет меня возвращаться повторно. Рекомендую всему миру, тем ценит высококачественный передышку и большие призы
What’s Going down i’m new to this, I stumbled upon this I have discovered
It absolutely helpful and it has aided me out loads.
I hope to contribute & aid other customers like its aided me.
Good job.
I feel that is one of the so much important info for me. And i am happy studying your
article. But wanna statement on few general things, The website style is ideal, the articles is in point of fact excellent : D.
Good job, cheers
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Добрый день всем! Мне бы хотелось сообщить своим опытом использования сайта мобильная версия казино лев. В этом месте вы отыщете широкий выбор развлечений, включая слоты, карточные игры и рулетку. Оформление сайта легкий в освоении, что совершает процесс игры удобным и приятным. В особенности порадовали скорые и достоверные методы внесения средств и вывода средств. Казино ещё предлагает превосходные бонусы для начинающих и постоянных игроков. Поддержка всегда на связи и подготовлена помочь решить любые проблемы. Но не забывайте о рисках, играя в игры на удачу. Везения всем участникам!
Добрый день всем! Желаю поделиться моим опытом пользования сайта казино драгон мани. Здесь вы найдёте широкий выбор развлечений, включая слоты, карточные игры и рулетку. Оформление сайта интуитивно понятный, что делает процесс игры комфортным и приятным. В особенности понравились быстрые и достоверные методы пополнения и вывода средств. Казино также предлагает превосходные бонусы для начинающих и постоянных игроков. Служба поддержки постоянно на контакте и подготовлена помочь упорядочить любые проблемы. Но не забывайте о рисках, играя в игры на удачу. Везения всем участникам!
Привет всем! Хочу поделиться своим опытом пользования сайта bounty casino рабочее зеркало. В этом месте вы обнаружите широкий ассортимент игр, с включением слоты, карточные игры и рулетку. Интерфейс сайта интуитивно понятный, что делает процесс игры удобным и приятным. В особенности порадовали быстрые и достоверные методы пополнения и вывода средств. Казино ещё предлагает отличные бонусы для новых и постоянных игроков. Служба поддержки постоянно на связи и подготовлена помочь упорядочить любые вопросы. Но помните о рисках, играя в азартные игры. Везения всем участникам!
I do agree with all the ideas you’ve introduced to your post.
They’re very convincing and can definitely work.
Still, the posts are very quick for beginners.
May you please extend them a little from next time?
Thank you for the post.
I think that is among the so much significant info for me.
And i am happy studying your article. However want to remark
on some general things, The site taste is perfect, the articles is truly nice : D.
Excellent process, cheers
Thanks for sharing your thoughts. I really appreciate your efforts and I am waiting for
your next post thanks once again.
I am really enjoying the theme/design of your web site.
Do you ever run into any web browser compatibility issues?
A handful of my blog readers have complained about
my site not working correctly in Explorer but looks great in Firefox.
Do you have any ideas to help fix this problem?
Simply wish to say your article is as amazing.
The clearness in your post is just spectacular and i could assume you are an expert on this
subject. Well with your permission allow me to grab your feed to keep up to date with
forthcoming post. Thanks a million and please carry on the rewarding work.
Thanks a lot for sharing this with all folks you really recognise what you are speaking
approximately! Bookmarked. Please additionally talk over with my site =).
We can have a hyperlink trade agreement among us
Because the admin of this web page is working, no hesitation very soon it will be renowned,
due to its feature contents.
Daarnaast willen spelers gewonnen bedragen zo snel mogelijk op hun bankrekening hebben staan.
Asking questions are genuinely pleasant thing if you are
not understanding anything totally, but this paragraph presents good understanding even.
We stumbled over here different page and thought I should check things out.
I like what I see so now i’m following you. Look forward
to looking over your web page again.
Take a look at my web page: Buy Tapentadol
Heya i’m for the primary time here. I came across this board and I find
It truly helpful & it helped me out much.
I’m hoping to give one thing again and help others like you
aided me.
I’m really enjoying the design and layout of your blog.
It’s a very easy on the eyes which makes it much more enjoyable for me to come
here and visit more often. Did you hire out a designer to create your theme?
Great work!
Votre vision de la digitalisation de l’artisanat est à la fois inspirante et réalisable.
Bravo!
Its like you read my mind! You appear to understand
so much about this, such as you wrote the e book in it or something.
I think that you could do with a few p.c. to force the message house
a bit, but instead of that, that is wonderful blog. An excellent read.
I’ll definitely be back.
I read this piece of writing completely concerning the difference of most recent and previous technologies,
it’s awesome article.
I read this article fully about the comparison of most recent
and preceding technologies, it’s remarkable article.
Amazing! Its truly amazing paragraph, I have got much clear idea on the topic of from this post.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Unibet heeft het meest uitgebreide aanbod van de Nederland met zowel casino (gokkasten, roulette, blackjack, stay casino), poker, bingo, en een sportsbook.
Hello, Neat post. There’s a problem with your website in web explorer, would check
this? IE nonetheless is the market leader and a large section of other
folks will miss your great writing due to this problem.
Just want to say your article is as astounding. The clarity
in your put up is just excellent and that i can assume you are
a professional on this subject. Fine with your permission allow me to grasp your feed to keep up to date with drawing close post.
Thank you a million and please keep up the gratifying work.
There’s certainly a lot to find out about this topic.
I love all of the points you have made.
My partner and I absolutely love your blog and find nearly all of your post’s tto
be just what I’m looking for. can you offer guest
writers to write content for you? I wouldn’t mind creating a post
or elaborating on a lot of the subjects you write related to here.
Again, awesome site!
My page :: brazilian wood supplement
I blog frequently and I genuinely appreciate your content.
This great article has truly peaked my interest.
I’m going to bookmark your website and keep checking for new information about once per week.
I subscribed to your RSS feed too.
I couldn’t refrain from commenting. Exceptionally well written!
Hello it’s me, I am also visiting this web site regularly, this website is
really pleasant and the visitors are in fact sharing pleasant thoughts.ラブドール 中古
Thanks , I’ve recently been looking for info about this subject for a while and yours is the best I’ve discovered so far.
But, what concerning the conclusion? Are you certain about the source?
Simply desire to say your article is as astonishing. The clearness in your
post is just nice and i can assume you are an expert on this subject.
Well with your permission allow me to grab your feed to keep up to date with forthcoming post.
Thanks a million and please carry on the rewarding work.
Quality articles is the secret to attract the users
to visit the web page, that’s what this site is providing.
Thank you. I value this!
Also visit my web site; https://May-massage.icu/daegu-massage
Hi there, I would like to subscribe for this web site to obtain most recent updates, therefore where can i do it please
help.
It’s very trouble-free to find out any topic on web as compared to textbooks, as I found this post at
this site.
This design is incredible! You certainly know how to keep a reader entertained.
Between your wit and your videos, I was almost moved to start my
own blog (well, almost…HaHa!) Excellent job. I really loved what you
had to say, and more than that, how you
presented it. Too cool!
An outstanding share! I’ve just forwarded this onto a friend who was doing
a little research on this. And he actually ordered me dinner due to the fact that I found it for
him… lol. So allow me to reword this…. Thanks for the meal!!
But yeah, thanks for spending time to discuss this issue here on your blog.
https://racoo.ir
I know this if off topic but I’m looking into starting my own blog and was
wondering what all is needed to get setup? I’m assuming having a blog like yours
would cost a pretty penny? I’m not very internet savvy
so I’m not 100% positive. Any suggestions or advice would be greatly appreciated.
Many thanks
When I originally commented I clicked the “Notify me when new comments are added” checkbox
and now each time a comment is added I get three emails with the same comment.
Is there any way you can remove people from that service? Many thanks!
I know this if off topic but I’m looking into starting my own weblog and
was curious what all is required to get setup? I’m assuming
having a blog like yours would cost a pretty penny?
I’m not very web smart so I’m not 100% sure.
Any recommendations or advice would be greatly appreciated.
Kudos
Please let me know if you’re looking for a writer
for your blog. You have some really good articles and I feel I would be a
good asset. If you ever want to take some of the load off, I’d absolutely love to write some content
for your blog in exchange for a link back to mine.
Please blast me an e-mail if interested. Regards!
Excellent beat ! I wish to apprentice even as you amend your
web site, how could i subscribe for a blog web site?
The account aided me a applicable deal. I were tiny bit acquainted of this your
broadcast provided shiny clear concept
Всем всем! Хочу поделиться своими ощущениями об интернет гэмблинг легзо. Это локация, где каждый геймер может отыскать свою счастье и насладиться волнительно игровым игрой. Ассортимент игр изумляет: от обычных слотов до модных видеоигр. Щедрые бонусы и регулярные акции превращают игру ещё еще более захватывающей. Удобный интерфейс и круглосуточная поддержка гарантируют комфортную игру в любое время. legzo casino – это не просто игровое заведение, это твой возможность на огромные выигрыши и незабываемые ощущения!
Всем привет! Хочу поделиться своими впечатлениями об онлайн казино старда казино официальный сайт. Это место, где все игрок может отыскать свою везение и получить удовольствие захватывающим игровым процессом. Ассортимент игровых процессов впечатляет: от обычных слотов до современных видеоигр. Большие бонусы и частые акции делают игру даже еще привлекательной. Комфортный интерфейс и постоянная поддержка обеспечивают приятную игру в любое время. casino starda – это не только игровой клуб, это ваш шанс на громадные достижения и незабываемые эмоции!
Привет привет! Хочу рассказать своими впечатлениями об цифровом игровом заведении Магнит слот казино официальный. Это локация, где каждый игрок может отыскать свою везение и получить удовольствие захватывающим игровым игрой. Ассортимент игр впечатляет: от традиционных слотов до современных видеоигр. Богатые бонусы и частые акции превращают игру даже более увлекательной. Комфортный интерфейс и 24/7 поддержка гарантируют приятную игру в всякое время. Магнитслотс – это не только игровой клуб, это твой шанс на огромные победы и памятные эмоции!
Здравствуйте всех! Хочу поделиться своими эмоциями об цифровом казино Официальный сайт казино Bollywood. Это локация, где все участник может обнаружить свою везение и получить удовольствие увлекательным игровым процессом. Выбор игр удивляет: от обычных слотов до актуальных видеоигр. Богатые бонусы и регулярные акции превращают игру даже еще привлекательной. Комфортный интерфейс и постоянная поддержка гарантируют приятную игру в любое время. bollywood casino зеркало – это не просто игровой клуб, это ваш возможность на крупные выигрыши и незабываемые эмоции!
Всем всем привет! Хочу поделиться своими впечатлениями об онлайн гэмблинг ramenbet casino. Это место, где любой участник может обнаружить свою удачу и насладиться захватывающим игровым процессом. Выбор игр впечатляет: от классических слотов до новейших видеоигр. Большие бонусы и частые акции делают игру даже еще более захватывающей. Удобный интерфейс и круглосуточная поддержка обеспечивают комфортную игру в всякое время. раменбет казино – это не только игровой клуб, это ваш возможность на большие победы и незабвенные ощущения!
Приветствую привет! Хочу поделиться своими отзывами об онлайн казино играть на деньги. Это заведение, где каждый игрок может найти свою фортуна и насладиться волнительно игровым процессом. Выбор игр удивляет: от традиционных слотов до модных видеоигр. Богатые бонусы и регулярные акции делают игру даже более заманчивой. Удобный интерфейс и постоянная поддержка обеспечивают комфортную игру в всякое время. Get X – это не просто гэмблинг, это твой возможность на огромные выигрыши и яркие ощущения!
Woah! I’m really digging the template/theme of this site.
It’s simple, yet effective. A lot of times it’s
very difficult to get that “perfect balance” between user friendliness and appearance.
I must say you’ve done a fantastic job with this.
Also, the blog loads extremely fast for me on Chrome. Exceptional Blog!
Having read this I believed it was rather enlightening.
I appreciate you taking the time and energy to put this article
together. I once again find myself spending way too much
time both reading and commenting. But so what, it was still worthwhile!
I do not even know how I ended up here, but I thought this post was great.
I do not know who you are but definitely you’re going to a famous blogger if you are not already 😉 Cheers!
Приветствую всем привет! Хочу поделиться своими ощущениями об веб казино казино Вулкан. Это место, где всякий игрок может обрести свою везение и насладиться волнительно игровым процессом. Ассортимент игр поражает: от традиционных слотов до современных видеоигр. Большие бонусы и постоянные акции превращают игру еще еще более привлекательной. Простой интерфейс и постоянная поддержка гарантируют удобную игру в всякое время. Игровые автоматы – это не только игровое заведение, это ваш возможность на крупные победы и памятные впечатления!
Does your blog have a contact page? I’m having a tough time locating it but, I’d like to shoot you an email.
I’ve got some recommendations for your blog you might be interested in hearing.
Either way, great site and I look forward to seeing it develop over time.
Heya i’m for the first time here. I found this
board and I find It really useful & it helped me out much.
I hope to give something back and aid others like you
aided me.
I absolutely love your blog and find almost all
of your post’s to be exactly I’m looking for.
can you offer guest writers to write content for you personally?
I wouldn’t mind composing a post or elaborating on a
number of the subjects you write in relation to here.
Again, awesome blog!
I am sure this piece of writing has touched all the internet
users, its really really good article on building up new website.
I really love your site.. Great colors & theme.
Did you build this site yourself? Please reply back as I’m
attempting to create my own site and want to know where you got this from or
just what the theme is named. Kudos!
Thanks a lot. Excellent information.
Hi there friends, fastidious article and pleasant arguments commented here,
I am in fact enjoying by these.
Link exchange is nothing else except it is only placing the other person’s web site link on your
page at appropriate place and other person will also do same in favor of you.
For latest news you have to pay a quick visit web and on the web I found
this web site as a finest web site for hottest updates.
I have learn a few good stuff here. Definitely
worth bookmarking for revisiting. I surprise how a lot attempt you place to create this kind of
fantastic informative web site.
Hey I am so excited I found your web site, I really
found you by mistake, while I was searching on Askjeeve for something else, Anyhow I am here now and
would just like to say thank you for a tremendous
post and a all round thrilling blog (I also love the theme/design), I don’t have time to browse it all at the moment but I have book-marked
it and also included your RSS feeds, so when I have time I
will be back to read a great deal more, Please do keep
up the fantastic work.
Hey there would you mind letting me know which webhost you’re utilizing?
I’ve loaded your blog in 3 completely different browsers and I
must say this blog loads a lot faster then most. Can you suggest a good internet hosting provider at a honest price?
Many thanks, I appreciate it!
Superb blog! Do you have any recommendations for aspiring writers?
I’m hoping to start my own blog soon but I’m a little lost on everything.
Would you advise starting with a free platform like WordPress or go for a paid
option? There are so many choices out there that I’m completely overwhelmed ..
Any tips? Thank you!
You have made some good points there. I checked on the net for additional information about the issue and found most individuals will go
along with your views on this site.
I’m very happy to find this website. I need to
to thank you for your time just for this fantastic read!!
I definitely liked every part of it and i also have you book-marked to look at new information in your web site.
If some one desires to be updated with latest technologies then he must be pay a quick visit this web site and be up to date
all the time.
Greetings from Los angeles! I’m bored to tears at work so I
decided to browse your blog on my iphone during lunch break.
I enjoy the information you provide here and can’t wait to take a look when I get home.
I’m amazed at how fast your blog loaded on my phone ..
I’m not even using WIFI, just 3G .. Anyhow, amazing blog!
Hey! Would you mind if I share your blog with my myspace group?
There’s a lot of folks that I think would really
enjoy your content. Please let me know. Thank you
Currently it looks like Movable Type is the preferred blogging platform out there right now.
(from what I’ve read) Is that what you are using on your blog?
Appreciation to my father who shared with
me concerning this blog, this blog is actually amazing.
Appreciating the time and effort you put into your
website and detailed information you present.
It’s nice to come across a blog every once in a while that isn’t the same outdated rehashed information. Great
read! I’ve bookmarked your site and I’m including your RSS feeds
to my Google account.
Hello, i think that i saw you visited my blog so i got here to return the desire?.I am trying to find
things to enhance my website!I suppose its ok to make use of some of your concepts!!
I was suggested this blog by my cousin. I’m not sure whether this post
is written by him as nobody else know such detailed about my trouble.
You are wonderful! Thanks!
Cet article offre une perspective profonde sur l’importance du digital pour les professionnels de la beauté.
Vos conseils sont non seulement innovants mais aussi immédiatement applicables.
Greetings! I know this is somewhat off topic but I was wondering which
blog platform are you using for this website? I’m getting fed
up of WordPress because I’ve had issues with hackers and I’m looking at options for another platform.
I would be fantastic if you could point me in the direction of a good platform.
Hey there! This post couldn’t be written any better! Reading this post reminds me of my good old room mate!
He always kept talking about this. I will forward this page to
him. Pretty sure he will have a good read. Thanks
for sharing!
Hello would you mind letting me know which webhost you’re working with?
I’ve loaded your blog in 3 completely different
internet browsers and I must say this blog loads a lot quicker then most.
Can you suggest a good internet hosting provider at a reasonable price?
Kudos, I appreciate it!
Hey excellent website! Does running a blog like this require a large amount of work?
I have virtually no expertise in computer programming however I
had been hoping to start my own blog in the near future. Anyways,
if you have any ideas or techniques for new blog owners please share.
I understand this is off topic nevertheless I simply needed to ask.
Thanks a lot!
Your style is very unique in comparison to other people I’ve read stuff from.
Thanks for posting when you have the opportunity, Guess I’ll just bookmark this web site.
This is really fascinating, You are an excessively skilled blogger.
I have joined your rss feed and sit up for in the hunt for
extra of your great post. Additionally, I have shared your site in my social networks
Howdy! I understand this is kind of off-topic but I needed to ask.
Does operating a well-established blog such as yours require a lot of work?
I’m completely new to writing a blog but I do write in my diary everyday.
I’d like to start a blog so I can easily share my personal experience
and views online. Please let me know if you have any recommendations
or tips for brand new aspiring bloggers. Thankyou!
You made some decent points there. I looked on the internet
to learn more about the issue and found most people
will go along with your views on this site.
Great blog here! Also your web site loads up very fast!
What web host are you using? Can I get your affiliate link to your host?
I wish my web site loaded up as quickly as yours lol
This post is truly a pleasant one it assists new the web viewers, who are wishing for blogging.
Hello it’s me, I am also visiting this website daily, this website
is actually nice and the users are truly sharing pleasant thoughts.
I have been browsing online more than 3 hours today, yet I never found any interesting article like yours.
It’s pretty worth enough for me. In my view, if all site owners and bloggers made good content as you did, the web will be much more
useful than ever before.
You are so awesome! I do not believe I have read a single
thing like this before. So good to find somebody with some unique thoughts on this issue.
Seriously.. thanks for starting this up. This web site is something that is needed on the internet,
someone with some originality!
My blog … custom led screen (Trent)
I think that is among the most important info for
me. And i am glad studying your article. However should statement on few general things, The web site style is great,
the articles is really great : D. Good process, cheers
Hi to all, it’s really a fastidious for me to visit this site, it contains precious Information.
I’ve learn a few excellent stuff here. Definitely value bookmarking for
revisiting. I wonder how so much effort you put to create this kind of great informative website.
Its like you read my mind! You appear to know so much about this,
like you wrote the book in it or something. I think that you can do with some pics to drive the message home a bit,
but other than that, this is wonderful blog. A great read.
I will certainly be back.
Simply desire to say your article is as surprising. The clarity in your post is simply great and i can assume you’re an expert on this subject.
Fine with your permission let me to grab your feed to keep updated with forthcoming post.
Thanks a million and please keep up the rewarding work.
We’re a gaggle of volunteers and opening a brand new scheme
in our community. Your web site provided us with useful information to work on. You have done an impressive
activity and our whole neighborhood will probably be
grateful to you.
Hi, all is going perfectly here and ofcourse every one is sharing information, that’s really excellent,
keep up writing.
Way cool! Some extremely valid points! I appreciate you penning this write-up plus the rest of
the website is really good.
Superb post however , I was wondering if you could write a litte more on this
subject? I’d be very thankful if you could elaborate a little bit further.
Cheers!
I’ve been exploring for a bit for any high quality articles or weblog posts
on this sort of space . Exploring in Yahoo I ultimately stumbled upon this website.
Studying this info So i am happy to show that I have a very just right uncanny feeling I found out just what I needed.
I such a lot surely will make sure to don?t forget this
website and provides it a look regularly.
Hi there, I think your blog may be having browser compatibility issues.
Whenever I look at your website in Safari, it
looks fine but when opening in IE, it has some overlapping issues.
I merely wanted to provide you with a quick heads up!
Apart from that, excellent site!
Here is my blog … led display screen panel
– Norma,
If some one desires to be updated with hottest technologies after that he must
be go to see this web site and be up to date daily.
I think that what you said was actually very logical. However, what
about this? suppose you wrote a catchier title? I am not suggesting
your content is not good., however what if you added something that makes people desire more?
I mean Valid Mountain Array – WebCodi is kinda plain. You might glance
at Yahoo’s home page and note how they create news titles to grab viewers interested.
You might add a video or a related pic or two to grab people excited about everything’ve got to
say. In my opinion, it would make your posts a little livelier.
hello there and thank you for your info – I have certainly picked up anything new from right
here. I did however expertise several technical issues using this web site, as I
experienced to reload the web site a lot of times previous to I could get
it to load properly. I had been wondering if your web host is OK?
Not that I’m complaining, but sluggish loading instances times will often affect your placement in google and
could damage your high quality score if ads and marketing with Adwords.
Well I’m adding this RSS to my email and could look out for a lot more of your respective exciting content.
Make sure you update this again very soon.
When I initially commented I clicked the “Notify me when new comments are added” checkbox and now each time a comment is added I get three e-mails
with the same comment. Is there any way you can remove me from that service?
Thanks a lot!
An interesting discussion is worth comment. I think that you ought to publish
more about this topic, it may not be a taboo subject but usually people do not discuss such subjects.
To the next! Best wishes!!
I am curious to find out what blog system you’re working with?
I’m having some small security issues with my latest site and I would like to find
something more safeguarded. Do you have any solutions?
You have made some good points there. I looked on the
web to find out more about the issue and found most people will go along with your views on this web
site.
Valuable info Kudos!
Hi, I do believe this is an excellent website. I stumbledupon it 😉 I am
going to revisit once again since I saved as a favorite it.
Money and freedom is the greatest way to change, may you
be rich and continue to guide other people.
You made some decent points there. I checked on the
web for additional information about the issue and found most people
will go along with your views on this web site.
I am regular visitor, how are you everybody?
This article posted at this web site is actually good.
I am sure this paragraph has touched all the internet users,
its really really fastidious article on building up new blog.
Wow! At last I got a website from where I can in fact obtain helpful data regarding my
study and knowledge.
Hmm it seems like your website ate my first comment (it
was super long) so I guess I’ll just sum it up what I wrote and say, I’m thoroughly enjoying your blog.
I too am an aspiring blog writer but I’m still new to everything.
Do you have any recommendations for rookie blog writers?
I’d really appreciate it.
امروز بیش از سه ساعت به صورت آنلاین
مرور بودهام، اما هرگز مقاله جالبی مانند مقاله شما پیدا نکردم.
این برای من به اندازه کافی ارزش دارد.
شخصا، اگر همه صاحبان وب و وبلاگ نویسان محتوای خوبی مانند
شما تولید کنند، اینترنت خیلی بیشتر با این
حال من به هیچ وجه مقاله جذاب مانند
شما را کشف کردم. این زیبا قیمت کافی است.
از نظر من، اگر همه مستران و وبلاگ نویسان درست محتوا را همانطور که محتواً درست
کردهاند. انجام داد، اینترنت
ممکن است خیلی بیشتر مفید از قبل.|
آها، این گفتگو مکالمه بسیار خوب است در
این صفحه وب، همه اینها را خوانده
ام، بنابراین در این زمان من نیز در این مکان نظر می دهم.|
مطمئنم این قطعه نوشته همه کاربران اینترنت را تحت تأثیر قرار داده است، واقعاً خوب پاراگراف در ایجاد وبلاگ جدید.|
وای، این پست خوب است، خواهر من
در حال تجزیه و تحلیل چنین چیزها است، بنابراین
به او بگویم را پیدا کردم، همانطور که من
این بند در این وب سایت.|
آیا وب سایت شما صفحه تماس دارد؟ من با
مشکلات سختی در مکان یابی
آن روبرو هستم، اما، میخواهم برای شما
یک ایمیل مشکل کنم. من برای وبلاگ شما ایده های خلاقانه دارم
که ممکن است علاقه مند به شنیدن آنها باشید.
در هر صورت، وبسایت فوقالعاده است و من مشتاقانه منتظر آن هستم که در طول زمان بهبود را ببینم.
در حال حاضر شجاعت را به دست آوردم تا به شما کمک
کنم. یک فریاد از هوستون Tx!
فقط می خواستم بگویم عالی کار را ادامه دهید!|
درود از لس آنجلس! من بی حوصلهدر محل
کار، بنابراین تصمیم گرفتم در زمان استراحت ناهار، وب سایت شما را در آیفون خود واقعاً دوست دارم و
نمیتوانم صبر کنم تا وقتی به خانه رسیدم نگاهی بیندازم.
من از بارگذاری {سریع} وبلاگ شما بر
روی موبایل من معجب هستم .. من حتی از WIFI استفاده نمی کنم، فقط 3G..
به هر حال، عالی وبلاگ!|
این مثل شما بیاموزید ذهن من!
شما {به نظر میرسد} میدانید خیلی درباره، مثل شما کتاب را
نوشتید. |e book} در آن یا چیزی.
معتقدم به سادگی میتوانید با
p.c. % انجام دهید تا فشار پیام خانه کمی، هر چند
وای، این همان چیزی بود که من به دنبالش بودم، چه
ماده! اینجا در این وبسایت وجود دارد، با
تشکر از مدیر این سایت. نوعی منطقه .
کاوش در یاهو من بالاخره به این سایت برخوردم.
خواندن این اطلاعات بنابراین من خوشحال} از بیان که دارم یک احساس فوقالعاده درست عجیب و غریب.
من مطمئنا حتما انجام خواهم
داد نه} این وبسایت را فراموش کنیداین
وبسایت را حذف کنید} نگاهی آن را
{به {یک نگاه ثابت} ارائه کنید} | ادامه دار |
بی امان} اساس|به طور منظم}.|
با خواندن این من باور کردم بسیار آموزنده}.
از اینکه این اطلاعات را این
مقاله را صرف بازدید سریع از وب سایت، این چیزی است که این وب
سایت ارائه می دهد.|
تبادل پیوند چیز دیگری نیست اما فقط پیوند وب سایت شخص دیگر را در مکان مناسب در صفحه خود قرار دهید.
و شخص دیگری نیز همین در حمایت شما را انجام خواهد
داد.|
من مطالب درباره دوستداران وبلاگ نویس را بسیار خوانده ام به جز این بند واقعاً یک خوشایند بند است، همینطور ادامه دهید.|
واقعا مهم نیست کسی میداند بعد به سایر افراد بستگی
دارد |بازدیدکنندگان} که آنها کمک خواهند کرد، بنابراین در اینجا روی میشود.|
شما حتما مطمئنا تخصص خود را در کاری که مینویسید ببینید.
بخش به حتی بیشتر نویسندگان پرشور مثل شما امیدوار است که {نمیترسند} از گفتن اعتقاد خود هراسی ندارند.
همیشه قلب خود را دنبال کنید.|
خوب پست. منروبهرو میشوم|در حال گذراندن |
خوب وبسایت دارید اینجا..
پیدا کردنش سخت است} با کیفیت بالا مانند شما این روزها.
راستش از افراد مثل شما قدردانی
می کنم! مراقب باش!!|
این وبلاگ توسط پسر عمویم پیشنهاد شد.
من مقاله یا بررسی درباره دوستداران وبلاگ نویس را بسیار خوانده ام اما این پست در واقع یک خوشایند بند
است، همینطور ادامه دهید. که آنها کمک، بنابراین در
اینجا اتفاق میافتد.|
شما حتما مطمئنا مهارتهای خود را در مقاله که مینویسید ببینید.
جهان به حتی بیشتر نویسندگان پرشور مثل شما امیدوار
است که {نمیترسند} از گفتن اعتقاد خود هراسی
ندارند. همیشه قلب خود را دنبال
کنید.|
خوب مقاله. باروبهرو میشوم|در
حال گذراندن |
عالی وبلاگ دارید اینجا.. پیدا کردنش سخت
است} عالی مانند شما امروز. واقعا
از امثال شما قدردانی می کنم!
مراقب باش!!|
این وبلاگ توسط پسر عمویم پیشنهاد شد.
در نظر بگیر. من به شما می گویم، من قطعاهمزمان با آزار همزمان |نگرانی|مسائل} که
آنها به وضوحدر مورد نگرانی هایی که به وضوح نمی دانند.
شما توانستید به بالا ضربه بزنید
و همچنین همه چیز را بدون داشتن عوارض جانبی مشخص کردید، مردم میتوانند روی وب سایت شما.|
وبلاگ عالی! من آن را در حین مرور در یاهو نیوز پیدا
کردم. آیا نکات در مورد نحوه قرار گرفتن
در لیست یاهو نیوز دارید؟ مدتی است که تلاش می کنم اما به نظر می رسد
هرگز به آنجا نرسیدم! سپاس فراوان|
عالی سایت. Lots of مفید اطلاعات در اینجا.
من آن را برای چند دوستان ارسال میکنم و همچنین در
خوشمزه به اشتراک میگذارند.
و مطمئنا، ممنونم عرق شما!|
فوق العاده پست، بسیار آموزنده. معجبم چرا برعکس متخصصان این بخش {نمیدانند} میفهمند.
نوشتن خود را باید ادامه.من مطمئن هستم}، شما پایگاه خوانندگان بسیار
بزرگی دارید!|
ضرب عالی ! وقتی وبسایت خود را اصلاح میکنید، میخواهم شاگردی
کنم، چگونه میتوان برای وبلاگ وبسایت مشترک شوم؟ حساب aided
روشن و شفاف ایده| آشنا بودم.
فوق العاده مسائل در مجموع، شما به سادگی بردید
یک آرم جدید. چه چیزی آیا شما توصیه میکنید درباره ارسال خود} چندروز در گذشته؟ آیا مثبت؟|
آیا اشکالی ندارید که چند از مقالات شما را تا زمانی که اعتبار و منابع
را به سایت شما ارائه میدهم نقل کنم؟ وبلاگ من
در بسیار یکسان توقف شماست و
بازدیدکنندگان من واقعا از
101 سود میبرند. بسیاری از اطلاعاتی که در اینجا {ارائه
میدهید}. لطفاً به من اطلاع دهید اگر این
بسیار خوب با شماست. ممنونم!|
با داشتن این همه محتوای نوشتاری آیا تا به حال
با مشکلات سرقت ادبی یا نقض حق چاپ مواجه شده اید؟ وب سایت من دارای محتوای کاملاً منحصر به فرد زیادی است که
من خودم یا خودم نویسنده} یا برون سپاری کرده ام، اما به نظر می
رسد بسیاری از آن ها هستند.
نمایش آن در سرتاسر اینترنت بدون موافق من.
آیا هیچ تکنیک برای کمک به
عالی {محصولات} از شما مرد. من مواظب باشید مطالب خود را قبلی به
و تو هستی فقط بسیار عالی. من در واقع آنچه را که تو خرید در اینجا،
مطمئنا دوست دارم تو بیان|گفتن} و راهی
که در آن بهوسیله شما ادعا میکنید.
میسازید آن را سرگرمکننده میکنید و
به آن ادامه میدهید مراقب
باشید تا آن را باهوش نگه دارید} .
من نمیتوانم صبر کنم تا از شما بخوانم خیلی بیشتر.
این است واقعا یک عالی وب سایت.|
اگر کسی نیاز دارد با بهروزترین فناوریها بهروز شود
بعد باید visit این وب سایت بروید و
روزانه به روز باشید.|
من معجب بودم که آیا تا به حال {به این فکر میکنید که}
ساختار سایت خود را تغییر دهید؟ بسیار خوب نوشته شده است؛
من عاشق چیزی هستم که باید بگویی اما شاید بتوانید کمی بیشتر در مسیر
محتوا قرار دهید تا مردم بتوانند بهتر با آن
ارتباط برقرار کنند. فقط برای داشتن یک یا دو
تصاویر متن بسیار زیادی دارید.
شاید بتوانید آن را بهتر از هم جدا کنید؟|
خیلی به سرعت این صفحه وب در
میان همه وبلاگنویسی و سایتسازی بینندگان، به دلیل اینکه پست است، خوب است}|
اگر کسی نیاز نظر متخصص مربوط راهاندازی
وبلاگ بعد از آن توصیه به او برای بازدید این صفحه، ادامه دهید خوب شغل.|
اجناس عالی از شما مرد. من مطالب شما را قبلاً درک کرده ام و شما فقط بسیار عالی} عالی.
من واقعاً آنچه را که در اینجا به
دست آوردهاید، واقعاً، واقعاً آنچه را که میگویی} و نحوه
بیان آن را دوست دارم. شما آن را سرگرم کننده میکنید و همچنان
مواظب هستید تا آن را عاقلانه نگه دارید.
من نمیتونم صبر کنم تا خیلی بیشتر از
شما بخونم. این واقعا یک برد فوق العاده استderful| فوق العاده} سایت.|
ضرب عالی ! من میخواهمدر حالی که وبسایت خود را اصلاح میکنید، چگونه میتوانم برای یک وبلاگ مشترک شوم.
سایت؟ حساب به من کمک کرد
یک معامله قابل قبول. من کمیآشنای} با
این پخش شما ارائه پر جنب و جوش شفاف
ایده|
معمولا من در وبلاگها آموختم مقاله میکنم، هر چند میخواهم بگویم که این نوشته بسیار مجبور تلاش و آن را انجام دهم!
طعم نوشتن شما مرا متعجب کرده است.
ممنونم، بسیار عالی مقاله.|
این وبسایت توسط پسر عمویم} پیشنهاد شد.
من دیگر مثبت آیا این پست نوشته شده است بسیار منظم
نوشته شده است. مطمئن شوید آن را نشانکگذاری
میکنم و برمیگردم تا از اطلاعات بیشتر مفید بیشتر.
بابت پست متشکرم. حتما برمی گردم.|
با تشکر برای شخصی را تخصص داشتم،
همانطور که بارگذاری مجدد وبسایت
بسیاری از بارها قبل از من میتوانستم آن را درست بارگیری کنم.
میدانستم که آیا میزبان
وب شما خوب است؟ نه اینکه من، اما زمانهای بارگیری گاهی روی قرارگیری شما در Google تأثیر میگذارد و میتواند به کیفیت در صورت تبلیغات و بازاریابی با Adwords.
به هر حال دارم این RSS را به e-mail خود
اضافه میکنم و میتوانم به دنبال
خیلی چیزهای دیگر جذاب شما باشم.
محتوای | هیجان انگیز}. مطمئن شوید این را دوباره خیلی
زود به روز کنید.|
آنچه من نمیفهمم این است که اگر حقیقت گفته
شود چگونه هستید نه واقعا خیلی بیشتر خوب-مورد علاقه از شما ممکن است در حال حاضر.
تو خیلی باهوشی. میدانید بنابراین بهطور قابلتوجهی ارتباط با
این موضوع، من را به نظر من در نظر بگیرید
آن را از {تصور کنید} زوایای بسیار زیاد|تعدادی|بسیاری از} متنوع.
مانند مردان و زنان به نظر نمیرسد درگیر به جز این
است چیزی کاری} برای انجام با
گاگای بانو! شخصی چیزهای بی نظیر است.
همیشه به آن رسیدگی کن!|
هورا! بالاخره من یک صفحه وب دریافت کردم که از آنجا توانم واقعاً |در واقع|درست|واقعاً} دریافت ارزشمند داده
درباره مطالعه و دانش من.|
وقتی کسی قطعه نوشتاری را می نویسد، ایده
یک کاربر را در ذهن خود کاربر می تواند آن را بداند.
بنابراین به همین دلیل است که این مقاله بینظیر است.
با تشکر! برای من، که این فرصت من دارم این عالی آموزنده مقاله را
اینجا در مسکن.|
سلام، همه اینجا صدا پیش میرود و البته همه اطلاعات را به اشتراک میگذارند، این واقعا خوب، به نوشتن ادامه دهید.|
یک سهم برجسته! من همین الان این را برای یک همکار که
بود یککوچکی تحقیق در مورد این.
و برای من در واقع سفارش داد صبحانه به این دلیل که برای او پیدا کردم روده بر
شدن از خنده. بنابراین {اجازه دهید} این
را دوباره بنویسم …. از شما برای وعده
غذایی!! اما بله، {تشکر} از
اینکه زمان را صرف بحث و گفتگو در مورد} این موضوع در اینجا در
وبلاگ.|
اوه این وبلاگ باعظمت خیلی دوست دارم پستهای شما را میخوانم|مطالعه میکنم.
{بمانید|به کار {خوب|عالی} ادامه دهید!
{میدانید|میفهمید|میدانید|میشناسید|از قبل میدانید}، {بسیاری|بسیاری از|خیلیها} {مردم هستند|افراد هستند|افراد} {شکار|جستجو|نگاه میکنند} {در اطراف|دور میگردند} برای این {اطلاعات|اطلاعات}،
{میتوانید|میتوانید} بسیار
به آنها {کمک|کمک کنید}. |
{سلام|هیا|هی|هی|روز بخیر|سلام|سلام|سلام|سلام|سلام}!
من می دانم که این تا حدی خارج از موضوع است {با این
حال،|با این وجود|اما|اما} فکر
می کردم بپرسم. آیا علاقه مند به
{تبادل|تجارت} پیوندها هستید یا
ممکن است مهمان {نوشتن|نویسنده} یک وبلاگ {مقاله|پست} باشید یا بالعکس؟ {وبسایت|سایت|وبلاگ} من {میگذرد|بحث میکند|آدرس|پوشش
میدهد}
constantly i used to read smaller articles or reviews that as
well clear their motive, and that is also happening with this post which I am reading now.
It’s in point of fact a great and helpful piece of information. I am
happy that you shared this helpful info
with us. Please keep us informed like this. Thanks for sharing.
Great blog! Is your theme custom made or did you download it
from somewhere? A theme like yours with a few simple tweeks would really make my blog shine.
Please let me know where you got your design.
Many thanks
Hello there! This blog post couldn’t be written any
better! Going through this article reminds me of my previous roommate!
He constantly kept talking about this. I am going to forward this information to him.
Pretty sure he’s going to have a very good read.
Thank you for sharing!
Hello there, just became aware of your blog through Google, and found that it’s really informative.
I am going to watch out for brussels. I will appreciate if
you continue this in future. A lot of people will be benefited from your writing.
Cheers!
I am regular visitor, how are you everybody? This article
posted at this website is really nice.
По количеству активных полос для начисления выигрышей и количеству барабанов.
200 линий выплат в современных слотах
открывают игрокам огромное
количество возможностей для выигрыша.
В то эпоха чисто в классических слотах обыкновенно используется небольшое численность
активных линий для выплат.
В отдельном разделе собраны интересные варианты настольных
азартных игр. Среди которых присутствуют: Для
игр с живыми дилерами уписывать отдельный раздел, в развлечениях этого типа игровым процессом управляет
реальный крупье. Здесь гемблеры
могут выступать единственно на реальные деньги, демонстрационный порядок тут не предусмотрен.
Все трансляции сопровождаются качественной
визуализацией. Для полного использования всех возможностей сайта Daddy
онлайн-казино и его мобильного приложения,
необходимо пролечь процесс регистрации.
Простая процедура состоит из нескольких шагов, включая заполнение
анкеты для создания игрового аккаунта.
Официальный сайт и мобильное приложение имеют удобный интерфейс,
что делает ориентацию новичка в них
легкой, а регистрацию без проблем для новых
пользователей. В онлайн казино Дэдди,
игровые автоматы непохожи дружок на друга, но при
этом они имеют схожие черты.
В каждом автомате присутствует игровое поле с изображением
барабанов и функциональных символов и панель управления.
С помощью специального блока кнопок, геймеры могут продавать параметры слота.
Wow, this piece of writing is good, my younger sister is analyzing such things, therefore I am going to tell her.
Hey there! I know this is kinda off topic but I was wondering which blog platform are you using for
this site? I’m getting fed up of WordPress because I’ve had issues with hackers and I’m looking at
options for another platform. I would be awesome if you could point me
in the direction of a good platform.
I do not even know how I ended up here, but I thought this post was great.
I don’t know who you are but definitely you’re going to a famous blogger if you are not already 😉 Cheers!
With havin so much content do you ever run into any issues of plagorism or copyright
violation? My site has a lot of unique content I’ve either authored myself or outsourced but it looks
like a lot of it is popping it up all over the internet without my authorization. Do you know any ways
to help reduce content from being stolen? I’d genuinely appreciate it.
دانستنی هایی در مورد دکمه xfan در کولر گازی و
کولر گازی 24000 جنرال گلد و کولر گازی nac
https://livemag.ir/?p=24670
Hello, Neat post. There’s an issue together with your site in web explorer, may check this?
IE nonetheless is the marketplace leader and a large component
of folks will miss your fantastic writing because of this problem.
You really make it seem so easy with your presentation but I find this topic to be actually
something that I think I would never understand.
It seems too complex and extremely broad for me. I’m looking forward for your next post, I’ll
try to get the hang of it!
Informative article, totally what I was looking for.
I’ve been surfing on-line more than three hours today, yet I by no
means discovered any attention-grabbing article like yours.
It’s lovely price sufficient for me. In my view, if all site owners and bloggers made excellent content material as you did,
the internet might be a lot more useful than ever before.
Appreciation to my father who shared with me about this blog, this web site
is genuinely remarkable.
With havin so much content and articles do you ever run into any issues of plagorism or copyright infringement?
My site has a lot of exclusive content I’ve either authored myself or
outsourced but it looks like a lot of it is popping it up all
over the internet without my permission. Do you know any techniques to help prevent content from being stolen? I’d really appreciate it.
Everyone loves what you guys are up too. This kind of clever work and exposure!
Keep up the good works guys I’ve added you guys to blogroll.
I’m not that much of a online reader to be honest but your sites really nice, keep it up!
I’ll go ahead and bookmark your site to come back later.
Cheers
I just couldn’t depart your site before suggesting that I really loved the
usual info an individual provide on your visitors?
Is going to be back incessantly to inspect new posts
penis enlargement
Hey there! This is my first visit to your blog!
We are a collection of volunteers and starting a new project in a community in the same niche.
Your blog provided us beneficial information to work on. You have done a extraordinary job!
Thank you for some other informative web site. The place else could I am getting that type of info written in such an ideal approach?
I have a mission that I am just now running on, and I have been at
the look out for such information.
I could not resist commenting. Well written!
Helpful info. Fortunate me I discovered your website
by accident, and I’m surprised why this coincidence did not
happened in advance! I bookmarked it.
Hiya! Quick question that’s entirely off topic. Do you
know how to make your site mobile friendly? My blog looks weird when viewing
from my iphone 4. I’m trying to find a theme or plugin that might be able to fix this problem.
If you have any suggestions, please share. Appreciate it!
Hi! This is my first visit to your blog! We are a collection of volunteers and starting a new project
in a community in the same niche. Your blog provided us valuable information to work on. You have done a
wonderful job!
Hello, Neat post. There’s a problem together with your web site in web explorer,
may check this? IE still is the marketplace leader and a huge element of folks will miss your magnificent writing because of this problem.
Heya i am for the first time here. I found this board and I find
It truly useful & it helped me out much. I hope to give
something back and help others like you helped me.
Hi! I just wanted to ask if you ever have any issues with hackers?
My last blog (wordpress) was hacked and I ended up losing a few months of hard work
due to no data backup. Do you have any solutions to stop hackers?
Hey would you mind letting me know which web host you’re utilizing?
I’ve loaded your blog in 3 completely different internet browsers
and I must say this blog loads a lot faster then most.
Can you suggest a good hosting provider at a reasonable price?
Cheers, I appreciate it!
I think everything typed was actually very reasonable.
But, what about this? what if you typed a catchier title?
I ain’t saying your information isn’t solid, but what if you
added something that grabbed a person’s attention? I mean Valid Mountain Array – WebCodi is kinda boring.
You might glance at Yahoo’s front page and watch how they create news titles to get people to open the links.
You might try adding a video or a related pic or two to grab people interested about everything’ve written. In my
opinion, it could make your posts a little bit more interesting.
Whats up this is somewhat of off topic but I was wondering if blogs
use WYSIWYG editors or if you have to manually code with HTML.
I’m starting a blog soon but have no coding skills so I wanted to get guidance from someone with experience.
Any help would be greatly appreciated!
I am regular visitor, how are you everybody? This piece of
writing posted at this website is actually nice.
Good way of describing, and fastidious post to get data concerning my presentation focus, which
i am going to present in institution of higher education.
Right now it sounds like Movable Type is the best blogging platform out there right
now. (from what I’ve read) Is that what you are using on your blog?
I needed to thank you for this excellent read!!
I absolutely enjoyed every little bit of
it. I have you book marked to check out new things
you post…
I am sure this paragraph has touched all the internet visitors, its really really nice piece of writing on building up new web site.
I’ve been exploring for a little bit for any high quality articles or blog posts on this sort of space .
Exploring in Yahoo I at last stumbled upon this web site.
Reading this information So i am glad to exhibit
that I have an incredibly excellent uncanny feeling
I found out just what I needed. I most without a doubt
will make certain to do not put out of your mind this web site and give it a look regularly.
I do not know if it’s just me or if everybody else experiencing problems with your website.
It appears like some of the written text within your content are running off the screen. Can someone else please comment and let me
know if this is happening to them too? This might be
a issue with my web browser because I’ve had this happen previously.
Many thanks
Fantastic material, With thanks!
You can certainly see your skills within the article you
write. The sector hopes for even more passionate writers such
as you who aren’t afraid to say how they believe.
At all times follow your heart.
Hello there, I discovered your blog by way of Google whilst searching for a related subject, your web site got
here up, it seems good. I have bookmarked it in my google bookmarks.
Hello there, simply was alert to your weblog thru Google, and found
that it is truly informative. I am gonna be careful for brussels.
I will be grateful when you continue this in future. Numerous
other folks will probably be benefited out of your writing.
Cheers!
We’re finances-pleasant – solely $10 a web page, considered one of the cheapest offers
on the market! It has grown through the years to be one of the most
important points of enterprise success.
Hey I know this is off topic but I was wondering if you
knew of any widgets I could add to my blog that automatically tweet
my newest twitter updates. I’ve been looking for a plug-in like this for quite
some time and was hoping maybe you would have some experience with something like
this. Please let me know if you run into anything.
I truly enjoy reading your blog and I look forward to your new updates.
Hey there, You’ve done a fantastic job. I’ll certainly digg it and personally suggest
to my friends. I’m sure they’ll be benefited from this web site.
Welcome to Flexy SMS Gateway, your trusted partner for budget-friendly and streamlined SMS solutions.
We focus on providing inexpensive SMS services, SMS gateway, and mass SMS options designed to
meet your business needs. Whether you’re looking to send marketing messages,
updates, or notifications, our robust SMS gateway ensures fast and secure delivery.
At FlexySMS, we understand the importance of uninterrupted communication. Our platform is designed to be user-friendly, flexible, and extremely reliable,
ensuring that your messages reach the intended recipients without any issues.
Leverage our SMS gateway to enhance customer engagement, optimize marketing campaigns, and optimize your communication processes.
Join the numerous businesses that trust FlexySMS for
their SMS needs and benefit from the difference of a service that’s
both affordable and reliable. Discover the
power of FlexySMS and take your business communication to the next level.
Hello! I know this is kinda off topic but I was wondering if you knew where
I could locate a captcha plugin for my comment form?
I’m using the same blog platform as yours and I’m having trouble finding one?
Thanks a lot!
Wow, this paragraph is nice, my younger sister is analyzing these kinds of
things, so I am going to convey her.
Check out my blog post … FitSpresso
If some one wishes to be updated with hottest technologies after that
he must be visit this website and be up to date all the time.
Hello! Allow me to start by saying my name – Roseline though I don’t
really like being called like that a lot of. What he
really enjoys doing is bottle tops collecting and that he is attempting make it a practice.
Some time ago she chose to live in North Carolina. Invoicing has
been my day job for years but I’ve already went
for another one.
موزیک باران
I’ve been exploring for a little for any high quality articles
or weblog posts in this kind of area . Exploring in Yahoo I ultimately stumbled upon this site.
Reading this info So i’m satisfied to express that I have a very just right uncanny
feeling I came upon just what I needed. I most for sure will make sure to do not
overlook this web site and give it a look regularly.
I am actually thankful to the owner of this website who has shared this
great paragraph at at this time.
Excellent, what a webpage it is! This web site gives helpful facts to
us, keep it up.
My brother suggested I might like this website.
He was entirely right. This post actually made my day.
You cann’t imagine just how much time I had spent for this info!
Thanks!
It’s a pity you don’t have a donate button! I’d certainly donate to this brilliant blog!
I suppose for now i’ll settle for bookmarking and adding your RSS feed to
my Google account. I look forward to new updates
and will talk about this website with my Facebook group.
Chat soon!
Hmm is anyone else having problems with the pictures on this
blog loading? I’m trying to find out if its a problem on my end or if it’s
the blog. Any feed-back would be greatly appreciated.
Aw, this was an exceptionally nice post. Taking the time and
actual effort to produce a very good article… but what can I say… I put things off a lot
and never seem to get nearly anything done.
It is the best time to make a few plans for the longer term and it is time to be happy.
I have learn this submit and if I could I wish to counsel you few attention-grabbing issues or suggestions.
Maybe you can write subsequent articles regarding this article.
I desire to learn even more issues approximately it!
bookmarked!!, I like your website!
Whoa! This blog looks exactly like my old one! It’s on a
entirely different topic but it has pretty much the same
page layout and design. Wonderful choice of colors!
It’s awesome in support of me to have a website,
which is beneficial designed for my knowledge.
thanks admin
Wonderful article! We are linking to this particularly great article on our site.
Keep up the great writing.
I’m really impressed with your writing skills and
also with the layout on your blog. Is this a
paid theme or did you modify it yourself? Either way keep up the excellent quality writing, it’s
rare to see a great blog like this one today.
It is also vital to understand your personal goal for proudly owning cryptocurrency.
Also, professional small companies cannot compete with
cash-laundering entrance companies that can afford to sell a
product for cheaper because their primary function is to clean cash, not flip
a profit. These companies could also be “front firms” that actually do provide a good or service however whose real function is to scrub
the launderer’s cash. This implies your cash may be saved separate from the rest of your funding portfolio.
On the socio-cultural end of the spectrum, successfully laundering cash means that
criminal exercise really does repay. This means more fraud,
more corporate embezzling (which implies extra workers shedding their pensions when the company
collapses), more medicine on the streets, extra drug-related crime, regulation-enforcement resources stretched past their means and a common lack of morale on the part
of reliable business people who don’t break the legislation and don’t make practically the income that
the criminals do. For the first time, I’ve
read by way of an entire article on CryptoEconomy and technlogy, and will perceive some part
of it.
My web site crypto online casino erstklassiger software
An impressive share! I have just forwarded this onto a friend who was conducting a little research on this.
And he actually bought me lunch because I stumbled upon it for him…
lol. So let me reword this…. Thanks for the meal!! But yeah, thanx for spending some time to discuss this matter here on your site.
I’m truly enjoying the design and layout of your site.
It’s a very easy on the eyes which makes it much more enjoyable for me to
come here and visit more often. Did you hire out
a developer to create your theme? Superb work!
Hi there, I enjoy reading through your article post.
I wanted to write a little comment to support you.
Superb, what a weblog it is! This weblog gives helpful facts to us, keep it up.
Hello! I know this is somewhat off-topic however I needed to ask.
Does building a well-established website like yours take a
large amount of work? I am brand new to blogging however
I do write in my diary every day. I’d like to start a blog so I can share my personal experience and thoughts online.
Please let me know if you have any recommendations or tips for
brand new aspiring blog owners. Appreciate it!
Hi my loved one! I wish to say that this post is awesome, great written and include almost all
vital infos. I’d like to see extra posts like this .
This blog was… how do I say it? Relevant!!
Finally I’ve found something that helped me. Thanks!
Thanks for a marvelous posting! I definitely enjoyed reading it, you can be a
great author. I will make certain to bookmark your blog and may come back later
in life. I want to encourage that you continue your great work, have a nice afternoon!
Nicely put. Appreciate it!
Hello There. I found your blog using msn. This is an extremely well written article.
I will be sure to bookmark it and come back to read
more of your useful information. Thanks for the post. I will certainly comeback.
Hello Dear, are you actually visiting this web site daily, if so
after that you will absolutely take nice knowledge.
I know this if off topic but I’m looking into starting my
own blog and was curious what all is needed
to get setup? I’m assuming having a blog like yours would cost a pretty penny?
I’m not very web savvy so I’m not 100% positive. Any tips or advice would be greatly appreciated.
Thank you
you are actually a just right webmaster. The web site loading speed
is incredible. It seems that you’re doing any distinctive trick.
Also, The contents are masterpiece. you’ve done a wonderful activity in this matter!
Hello i am kavin, its my first occasion to commenting anywhere, when i read this
piece of writing i thought i could also create comment due to this
sensible post.
Thanks for sharing your thoughts on udin777. Regards